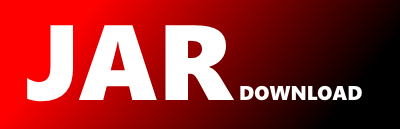
com.jongsoft.highchart.series.Series Maven / Gradle / Ivy
/*
* The MIT License
*
* Copyright 2016 Jong Soft.
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in
* all copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
* THE SOFTWARE.
*/
package com.jongsoft.highchart.series;
import com.jongsoft.highchart.AbstractHighchart;
import com.jongsoft.highchart.axis.Marker;
import com.jongsoft.highchart.common.CursorType;
import com.jongsoft.highchart.common.GraphColor;
import com.jongsoft.highchart.common.SeriesType;
import java.util.List;
/**
* The actual series to append to the chart. In addition to the members listed below, any member of the plotOptions for that specific type of plot can be added
* to a series individually. For example, even though a general lineWidth is specified in plotOptions.series, an individual lineWidth can be specified for each
* series.
*/
public abstract class Series {
private Boolean allowPointSelect;
private Boolean animation;
private GraphColor color;
private Number cropThreshold;
private CursorType cursor;
private List data;
private DataLabels dataLabels;
private Boolean enableMouseTracking;
private String name;
private SeriesType type;
private Marker marker;
private Number xAxis;
private Number yAxis;
private Number zIndex;
private String zoneAxis;
protected Series(SeriesType type) {
this.type = type;
}
protected void setType(SeriesType type) {
this.type = type;
}
/**
* Enable or disable the initial animation when a series is displayed. The animation can also be set as a configuration object. Please note that this option
* only applies to the initial animation of the series itself.
*/
public T setAnimation(Boolean animation) {
this.animation = animation;
return self();
}
/**
* The name of the series as shown in the legend, tooltip etc.
*/
public T setName(String name) {
this.name = name;
return self();
}
/**
* Allow this series' points to be selected by clicking on the markers, bars or pie slices.
*
* Default: false.
*/
public T setAllowPointSelect(Boolean allowPointSelect) {
this.allowPointSelect = allowPointSelect;
return self();
}
/**
* The main color or the series. In line type series it applies to the line and the point markers unless otherwise specified. In bar type series it applies
* to the bars unless a color is specified per point.
*
* The default value is pulled from the {@link AbstractHighchart#getColors()} array.
*/
public T setColor(GraphColor color) {
this.color = color;
return self();
}
/**
* When the series contains less points than the crop threshold, all points are drawn, event if the points fall outside the visible plot area at the current
* zoom. The advantage of drawing all points (including markers and columns), is that animation is performed on updates. On the other hand, when the series
* contains more points than the crop threshold, the series data is cropped to only contain points that fall within the plot area.
*
* The advantage of cropping away invisible points is to increase performance on large series.
*
* Default: 300.
*/
public T setCropThreshold(Number cropThreshold) {
this.cropThreshold = cropThreshold;
return self();
}
/**
* You can set the cursor to "pointer" if you have click events attached to the series, to signal to the user that the points and lines can be clicked.
*/
public T setCursor(CursorType cursor) {
this.cursor = cursor;
return self();
}
/**
* Enable or disable the mouse tracking for a specific series. This includes point tooltips and click events on graphs and points. For large datasets it
* improves performance.
*
* Default: true
*/
public T setEnableMouseTracking(Boolean enableMouseTracking) {
this.enableMouseTracking = enableMouseTracking;
return self();
}
/**
* Add a point to the {@link Series} which will translate into one point on the chart.
*/
public T addPoint(SeriesPoint point) {
if (data == null) {
data = new PointList();
}
data.add(point);
return self();
}
/**
* When using dual or multiple x axes, this number defines which xAxis the particular series is connected to. It refers to either the axis id or the index
* of the axis in the xAxis array, with 0 being the first.
*/
public T setXAxis(Number xAxis) {
this.xAxis = xAxis;
return self();
}
/**
* When using dual or multiple y axes, this number defines which yAxis the particular series is connected to. It refers to either the axis id or the index
* of the axis in the yAxis array, with 0 being the first
*/
public T setYAxis(Number yAxis) {
this.yAxis = yAxis;
return self();
}
/**
* Define the visual z index of the series.
*/
public T setZIndex(Number zIndex) {
this.zIndex = zIndex;
return self();
}
/**
* Defines the Axis on which the zones are applied.
*
* Default: y
*/
public T setZoneAxis(String zoneAxis) {
this.zoneAxis = zoneAxis;
return self();
}
protected abstract T self();
public DataLabels getDataLabels() {
if (dataLabels == null) {
dataLabels = new DataLabels(self());
}
return dataLabels;
}
public Marker getMarker() {
if (marker == null) {
marker = new Marker<>(self());
}
return marker;
}
public SeriesType getType() {
return type;
}
public List getData() {
return data;
}
public Boolean getAnimation() {
return animation;
}
public String getName() {
return name;
}
public Boolean getAllowPointSelect() {
return allowPointSelect;
}
public GraphColor getColor() {
return color;
}
public Number getCropThreshold() {
return cropThreshold;
}
public CursorType getCursor() {
return cursor;
}
public Number getxAxis() {
return xAxis;
}
public Number getyAxis() {
return yAxis;
}
public Boolean getEnableMouseTracking() {
return enableMouseTracking;
}
}