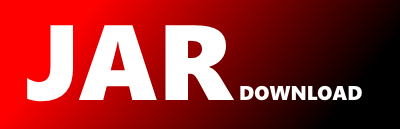
com.jparams.store.UnmodifiableStore Maven / Gradle / Ivy
package com.jparams.store;
import java.util.Collection;
import java.util.Iterator;
import java.util.Objects;
import java.util.Optional;
import com.jparams.store.comparison.ComparisonPolicy;
import com.jparams.store.index.Index;
import com.jparams.store.index.IndexException;
/**
* Implementation of a store that cannot be modified
*
* @param value type
*/
public class UnmodifiableStore implements Store
{
private final Store store;
public UnmodifiableStore(final Store store)
{
this.store = store;
}
@Override
public Index multiIndex(final String indexName, final KeyProvider, V> keyProvider) throws IndexException
{
throw new UnsupportedOperationException();
}
@Override
public Index multiIndex(final KeyProvider, V> keyProvider, final ComparisonPolicy comparisonPolicy) throws IndexException
{
throw new UnsupportedOperationException();
}
@Override
public Index multiIndex(final KeyProvider, V> keyProvider) throws IndexException
{
throw new UnsupportedOperationException();
}
@Override
public Index index(final String indexName, final KeyProvider keyProvider, final ComparisonPolicy comparisonPolicy) throws IndexException
{
throw new UnsupportedOperationException();
}
@Override
public Index index(final String indexName, final KeyProvider keyProvider) throws IndexException
{
throw new UnsupportedOperationException();
}
@Override
public Index index(final KeyProvider keyProvider, final ComparisonPolicy comparisonPolicy) throws IndexException
{
throw new UnsupportedOperationException();
}
@Override
public Index index(final KeyProvider keyProvider) throws IndexException
{
throw new UnsupportedOperationException();
}
@Override
public void removeAllIndexes()
{
throw new UnsupportedOperationException();
}
@Override
public Optional> findIndex(final String indexName)
{
return store.findIndex(indexName);
}
@Override
public boolean addAll(final V[] items) throws IndexException
{
throw new UnsupportedOperationException();
}
@Override
public Store synchronizedStore()
{
return store.synchronizedStore().unmodifiableStore();
}
@Override
public Index multiIndex(final String indexName, final KeyProvider, V> keyProvider, final ComparisonPolicy comparisonPolicy) throws IndexException
{
throw new UnsupportedOperationException();
}
@Override
public Index getIndex(final String indexName)
{
return store.getIndex(indexName);
}
@Override
public Collection> getIndexes()
{
return store.getIndexes();
}
@Override
public boolean removeIndex(final Index index)
{
throw new UnsupportedOperationException();
}
@Override
public boolean removeIndex(final String indexName)
{
throw new UnsupportedOperationException();
}
@Override
public void reindex()
{
throw new UnsupportedOperationException();
}
@Override
public void reindex(final Collection items)
{
throw new UnsupportedOperationException();
}
@Override
public void reindex(final V item)
{
throw new UnsupportedOperationException();
}
@Override
public Store unmodifiableStore()
{
return this;
}
@Override
public int size()
{
return store.size();
}
@Override
public boolean isEmpty()
{
return store.isEmpty();
}
@Override
public boolean contains(final Object obj)
{
return store.contains(obj);
}
@Override
public Iterator iterator()
{
return new UnmodifiableIterator<>(store.iterator());
}
@Override
public Object[] toArray()
{
return store.toArray();
}
@Override
public T1[] toArray(final T1[] array)
{
return store.toArray(array);
}
@Override
public boolean add(final V item)
{
throw new UnsupportedOperationException();
}
@Override
public boolean remove(final Object o)
{
throw new UnsupportedOperationException();
}
@Override
public boolean containsAll(final Collection> collection)
{
return store.containsAll(collection);
}
@Override
public boolean addAll(final Collection extends V> collection)
{
throw new UnsupportedOperationException();
}
@Override
public boolean removeAll(final Collection> collection)
{
throw new UnsupportedOperationException();
}
@Override
public boolean retainAll(final Collection> collection)
{
throw new UnsupportedOperationException();
}
@Override
public void clear()
{
throw new UnsupportedOperationException();
}
@Override
public Store copy()
{
return store.copy();
}
@Override
public boolean equals(final Object obj)
{
if (this == obj)
{
return true;
}
if (obj == null || getClass() != obj.getClass())
{
return false;
}
final UnmodifiableStore> that = (UnmodifiableStore>) obj;
return Objects.equals(store, that.store);
}
@Override
public int hashCode()
{
return Objects.hash(store);
}
@Override
public String toString()
{
return store.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy