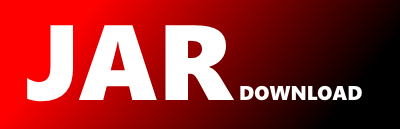
com.jparams.store.index.AbstractIndex Maven / Gradle / Ivy
package com.jparams.store.index;
import java.util.Collection;
import java.util.Objects;
import java.util.Set;
import java.util.stream.Collectors;
import com.jparams.store.KeyProvider;
import com.jparams.store.comparison.ComparisonPolicy;
import com.jparams.store.reference.Reference;
public abstract class AbstractIndex implements Index
{
private final String name;
private final KeyProvider, V> keyProvider;
private final ComparisonPolicy comparisonPolicy;
protected AbstractIndex(final String name, final KeyProvider, V> keyProvider, final ComparisonPolicy comparisonPolicy)
{
this.name = name;
this.keyProvider = keyProvider;
this.comparisonPolicy = comparisonPolicy;
}
protected Set
© 2015 - 2025 Weber Informatics LLC | Privacy Policy