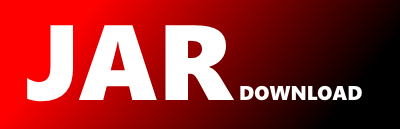
com.jparams.store.SynchronizedStore Maven / Gradle / Ivy
package com.jparams.store;
import java.util.Collection;
import java.util.Iterator;
import java.util.List;
import java.util.Optional;
import java.util.function.Predicate;
import java.util.stream.Collectors;
import com.jparams.store.index.Index;
import com.jparams.store.index.IndexDefinition;
import com.jparams.store.index.IndexException;
import com.jparams.store.index.KeyMapper;
import com.jparams.store.index.SynchronizedIndex;
import com.jparams.store.index.reducer.Reducer;
import com.jparams.store.query.Query;
/**
* Synchronized (thread-safe) store backed by given store.
*
* @param value type
*/
public class SynchronizedStore implements Store
{
private final Store store;
private final Object mutex;
public SynchronizedStore(final Store store)
{
this.store = store;
this.mutex = this;
}
@Override
public Index getIndex(final String indexName)
{
final Index index;
synchronized (mutex)
{
final Index found = store.getIndex(indexName);
index = found == null ? null : new SynchronizedIndex<>(found, mutex);
}
return index;
}
@Override
public Collection> getIndexes()
{
final List> indexes;
synchronized (mutex)
{
indexes = store.getIndexes().stream().map(index -> new SynchronizedIndex<>(index, mutex)).collect(Collectors.toList());
}
return indexes;
}
@Override
public void removeAllIndexes()
{
synchronized (mutex)
{
store.removeAllIndexes();
}
}
@Override
public Optional> findIndex(final String indexName)
{
final Optional> optionalIndex;
synchronized (mutex)
{
optionalIndex = store.findIndex(indexName).map(index -> new SynchronizedIndex<>(index, mutex));
}
return optionalIndex;
}
@Override
public boolean removeIndex(final Index index)
{
final boolean removed;
synchronized (mutex)
{
if (index instanceof SynchronizedIndex)
{
removed = store.removeIndex(((SynchronizedIndex) index).getIndex());
}
else
{
removed = store.removeIndex(index);
}
}
return removed;
}
@Override
public boolean removeIndex(final String indexName)
{
final boolean removed;
synchronized (mutex)
{
removed = store.removeIndex(indexName);
}
return removed;
}
@Override
public Index index(final String indexName, final IndexDefinition indexDefinition) throws IndexException
{
final Index index;
synchronized (mutex)
{
index = store.index(indexName, indexDefinition);
}
return index;
}
@Override
public Index index(final IndexDefinition indexDefinition) throws IndexException
{
final Index index;
synchronized (mutex)
{
index = store.index(indexDefinition);
}
return index;
}
@Override
public Index index(final String indexName, final KeyMapper keyMapper) throws IndexException
{
final Index index;
synchronized (mutex)
{
index = store.index(indexName, keyMapper);
}
return index;
}
@Override
public Index index(final KeyMapper keyMapper) throws IndexException
{
final Index index;
synchronized (mutex)
{
index = store.index(keyMapper);
}
return index;
}
@Override
public Index index(final String indexName, final KeyMapper keyMapper, final Reducer reducer) throws IndexException
{
final Index index;
synchronized (mutex)
{
index = store.index(indexName, keyMapper, reducer);
}
return index;
}
@Override
public Index index(final KeyMapper keyMapper, final Reducer reducer) throws IndexException
{
final Index index;
synchronized (mutex)
{
index = store.index(keyMapper, reducer);
}
return index;
}
@Override
public List get(final String indexName, final Object key, final Integer limit)
{
final List results;
synchronized (mutex)
{
results = store.get(indexName, key, limit);
}
return results;
}
@Override
public List get(final String indexName, final Object key)
{
final List results;
synchronized (mutex)
{
results = store.get(indexName, key);
}
return results;
}
@Override
public V getFirst(final String indexName, final Object key)
{
final V result;
synchronized (mutex)
{
result = store.getFirst(indexName, key);
}
return result;
}
@Override
public Optional findFirst(final String indexName, final Object key)
{
final Optional result;
synchronized (mutex)
{
result = store.findFirst(indexName, key);
}
return result;
}
@Override
public List get(final Query query, final Integer limit)
{
final List results;
synchronized (mutex)
{
results = store.get(query, limit);
}
return results;
}
@Override
public List get(final Query query)
{
final List results;
synchronized (mutex)
{
results = store.get(query);
}
return results;
}
@Override
public V getFirst(final Query query)
{
final V result;
synchronized (mutex)
{
result = store.getFirst(query);
}
return result;
}
@Override
public Optional findFirst(final Query query)
{
final Optional result;
synchronized (mutex)
{
result = store.findFirst(query);
}
return result;
}
@Override
public void reindex()
{
synchronized (mutex)
{
store.reindex();
}
}
@Override
public void reindex(final Collection items)
{
synchronized (mutex)
{
store.reindex(items);
}
}
@Override
public void reindex(final V item)
{
synchronized (mutex)
{
store.reindex(item);
}
}
@Override
public Store copy()
{
final Store copy;
synchronized (mutex)
{
copy = store.copy();
}
return copy;
}
@Override
public int size()
{
final int size;
synchronized (mutex)
{
size = store.size();
}
return size;
}
@Override
public boolean isEmpty()
{
final boolean empty;
synchronized (mutex)
{
empty = store.isEmpty();
}
return empty;
}
@Override
public boolean contains(final Object obj)
{
final boolean contains;
synchronized (mutex)
{
contains = store.contains(obj);
}
return contains;
}
@Override
public Iterator iterator()
{
return store.iterator();
}
@Override
public Object[] toArray()
{
final Object[] array;
synchronized (mutex)
{
array = store.toArray();
}
return array;
}
@Override
public T1[] toArray(final T1[] array)
{
final T1[] toArray;
synchronized (mutex)
{
toArray = store.toArray(array);
}
return toArray;
}
@Override
public boolean add(final V item)
{
final boolean result;
synchronized (mutex)
{
result = store.add(item);
}
return result;
}
@Override
public boolean remove(final Object obj)
{
final boolean result;
synchronized (mutex)
{
result = store.remove(obj);
}
return result;
}
@Override
public boolean containsAll(final Collection> collection)
{
final boolean result;
synchronized (mutex)
{
result = store.containsAll(collection);
}
return result;
}
@Override
public boolean addAll(final Collection extends V> collection)
{
final boolean result;
synchronized (mutex)
{
result = store.addAll(collection);
}
return result;
}
@Override
public boolean addAll(final V[] items) throws IndexException
{
final boolean result;
synchronized (mutex)
{
result = store.addAll(items);
}
return result;
}
@Override
public boolean removeAll(final Collection> collection)
{
final boolean result;
synchronized (mutex)
{
result = store.removeAll(collection);
}
return result;
}
@Override
public boolean removeIf(final Predicate super V> filter)
{
final boolean result;
synchronized (mutex)
{
result = store.removeIf(filter);
}
return result;
}
@Override
public boolean retainAll(final Collection> collection)
{
final boolean result;
synchronized (mutex)
{
result = store.retainAll(collection);
}
return result;
}
@Override
public void clear()
{
synchronized (mutex)
{
store.clear();
}
}
public Store getStore()
{
return store;
}
@Override
public Store synchronizedStore()
{
return this;
}
@Override
public String toString()
{
return store.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy