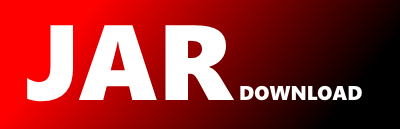
com.jpattern.core.command.ACommand Maven / Gradle / Ivy
package com.jpattern.core.command;
import com.jpattern.core.IProvider;
import com.jpattern.core.NullProvider;
import com.jpattern.core.command.ICommand;
import com.jpattern.core.command.ICommandResult;
import com.jpattern.logger.ILogger;
import com.jpattern.shared.result.ErrorMessage;
/**
*
* @author Francesco Cina'
*
* 27/feb/2011
*/
public abstract class ACommand extends ICommand implements Runnable {
private static final long serialVersionUID = 1L;
private static final String RUNTIME_EXEC_ERROR_PROPERTY_MESSAGE = "generic.exec.runtime.error";
private static final String RUNTIME_ROLLBACK_ERROR_PROPERTY_MESSAGE = "generic.exec.runtime.error";
private IProvider provider;
private ICommand previousCommand;
private boolean executed = false;
private ILogger logger;
private IChainStrategy chainStrategy;
private ICommandResult result;
public ACommand(ICommand previousCommand) {
this.previousCommand = previousCommand;
}
@Override
public final ICommandResult exec() {
return exec(new ConditionalCommandExecutor());
}
@Override
public final ICommandResult exec(ICommandExecutor aCommandExecutor) {
ICommandResult result = prepareCommandResult();
doExec(aCommandExecutor, result);
aCommandExecutor.getChainStrategy().globalExecEnd( this , result);
return result;
}
@Override
final ICommandResult prepareCommandResult() {
ICommandResult result = getPreviousCommand().prepareCommandResult();
result.commandStartExecution(this);
return result;
}
@Override
final void doExec(ICommandExecutor aCommandExecutor, ICommandResult result) {
this.chainStrategy = aCommandExecutor.getChainStrategy();
this.result = result;
getPreviousCommand().doExec(aCommandExecutor, result);
if (aCommandExecutor.executeNext(result)) {
aCommandExecutor.addCommandToPool(this);
}
}
@Override
void doGlobalRollback(ICommandResult result){
doLocalRollback(result);
result.commandEndExecution(this);
getPreviousCommand().doGlobalRollback(result);
}
void doLocalRollback(ICommandResult result) {
if (executed) {
try {
internalRollBack(result);
setExecuted(false);
} catch (RuntimeException e) {
getLogger().error("run", "RuntimeException thrown", e);
result.addErrorMessage(new ErrorMessage(this.getClass().getSimpleName(), RUNTIME_ROLLBACK_ERROR_PROPERTY_MESSAGE));
}
}
}
@Override
public final void visit(IProvider provider) {
getPreviousCommand().visit(provider);
this.provider = provider;
}
@Override
public final void run() {
ICommandResult localresult = new CommandResult();
try {
result(localresult);
} catch (RuntimeException e) {
getLogger().error("run", "RuntimeException thrown", e);
localresult.addErrorMessage(new ErrorMessage(this.getClass().getSimpleName(), RUNTIME_EXEC_ERROR_PROPERTY_MESSAGE));
} finally {
setExecuted(true);
try {
chainStrategy.localExecEnd(this, localresult, result);
} catch (RuntimeException e) {
getLogger().error("run", "RuntimeException thrown", e);
result.addErrorMessage(new ErrorMessage(this.getClass().getSimpleName(), RUNTIME_ROLLBACK_ERROR_PROPERTY_MESSAGE));
}
result.commandEndExecution(this);
}
}
protected final ILogger getLogger() {
if (logger==null) {
logger = getProvider().getLoggerService().logger(this.getClass());
}
return logger;
}
protected abstract void result(ICommandResult result);
protected abstract void internalRollBack(ICommandResult result);
protected ICommand getPreviousCommand() {
if (previousCommand==null) {
previousCommand = new NullCommand();
}
return previousCommand;
}
protected IProvider getProvider() {
if (provider==null) {
provider = new NullProvider();
}
return provider;
}
boolean isExecuted() {
return executed;
}
void setExecuted(boolean executed) {
this.executed = executed;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy