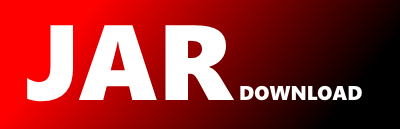
com.jpattern.core.command.CommandResult Maven / Gradle / Ivy
package com.jpattern.core.command;
import java.util.Iterator;
import java.util.List;
import java.util.Vector;
import com.jpattern.core.command.ICommand;
import com.jpattern.shared.result.IErrorMessage;
/**
*
* @author Francesco Cina'
*
* 27/feb/2011
*/
public class CommandResult extends ICommandResult {
private static final long serialVersionUID = 1L;
private List commandInExecutionList = new Vector();
private List errorMessages;
public CommandResult() {
errorMessages = new Vector();
}
@Override
public final synchronized boolean isExecutionEnd() {
return commandInExecutionList.size()==0;
}
@Override
public final synchronized void waitExecutionEnd() throws InterruptedException {
if (!isExecutionEnd()) {
wait();
}
}
@Override
protected final synchronized void commandStartExecution(ICommand aCommand) {
commandInExecutionList.add(aCommand);
}
@Override
protected final synchronized void commandEndExecution(ICommand aCommand) {
commandInExecutionList.remove(aCommand);
if (isExecutionEnd()) {
notifyAll();
}
}
@Override
protected final synchronized void removeAllCommands() {
commandInExecutionList.clear();
if (isExecutionEnd()) {
notifyAll();
}
}
@Override
public final synchronized List getErrorMessages() {
if ( errorMessages == null ) {
errorMessages = new Vector();
}
return errorMessages;
}
@Override
public final synchronized void addErrorMessage(IErrorMessage errorMessage) {
getErrorMessages().add(errorMessage);
}
@Override
public synchronized boolean isValid() {
return getErrorMessages().size() == 0;
}
@Override
public synchronized String asString() {
StringBuffer buffer = new StringBuffer();
buffer.append("[ \n");
buffer.append("{ isValid = " + isValid() + " } \n");
// buffer.append("{ returnObject = " + returnedObject() + " } \n");
buffer.append("{ errormessages = \n");
for (Iterator iter = errorMessages.iterator(); iter.hasNext();) {
IErrorMessage msg = iter.next();
buffer.append("( " + msg.getName() + " : " + msg.getMessage());
int len = msg.getParameters().size();
for (int i = 0; i < len; i++) {
buffer.append(" " + msg.getParameters().get(i) + " ");
}
buffer.append(" )");
}
buffer.append(" }\n");
buffer.append(" ] ");
return buffer.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy