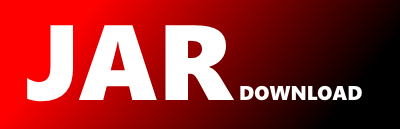
com.jpattern.core.command.ICommand Maven / Gradle / Ivy
package com.jpattern.core.command;
import com.jpattern.core.IProvider;
import com.jpattern.core.exception.NullProviderException;
import com.jpattern.logger.ILogger;
import com.jpattern.logger.SystemOutLoggerFactory;
/**
*
* @author Francesco Cina'
*
* 11/set/2011
*/
public abstract class ICommand {
private ICommandExecutor commandExecutor;
private T provider;
private ILogger logger = null;
ICommand() {}
/**
* This method launch the execution of the command (or chain of commands) using the default
* default Executor and catching every runtime exception.
* This command is the same of:
* exec(provider, true);
* @return the result of the execution
*/
public final ICommandResult exec(T provider) {
return exec(provider, true);
}
/**
* This method launch the execution of the command (or chain of commands) using the default
* default Executor.
* This command is the same of:
* exec(provider, new DefaultCommandExecutor(), catchRuntimeException);
* @param catchRuntimeException whether catch or propagate a RuntimeException
* @return the result of the execution
*/
public final ICommandResult exec(T provider, boolean catchRuntimeException) {
return exec(provider, new DefaultCommandExecutor(), catchRuntimeException);
}
/**
* This method launch the execution of the command (or chain of commands).
* Every command in the chain will be managed by an ICommandExecutor object.
* This command is the same of:
* exec(commandExecutor, true);
* @param aCommandExecutor the pool in which the command will runs
* @return the result of the execution
*/
public final ICommandResult exec(T provider, ICommandExecutor commandExecutor) {
return exec(provider, commandExecutor, true);
}
/**
* This method launch the execution of the command (or chain of commands).
* Every command in the chain will be managed by an ICommandExecutor object.
* If the catchRuntimeException is true every RuntimeException will be caught and a new ErrorMessage in the
* error list is created; otherwise the Runtime Exception will be propagated to the command caller.
* @param aCommandExecutor the pool in which the command will runs
* @param catchRuntimeException whether catch or propagate a RuntimeException
* @return the result of the execution
*/
public final ICommandResult exec(T provider, ICommandExecutor commandExecutor, boolean catchRuntimeException) {
visit(provider);
return exec( commandExecutor, catchRuntimeException, new CommandResult());
}
/**
* This method launch the rollback of the command execution (or chain of commands) using the default
* default Executor and catching every runtime exception.
* The rollback is effectively performed only if the command has been executed with a positive result, otherwise
* the command is intended as "not executed" then no rollback will be performed.
* This command is the same of:
* rollback(provider, true);
* @return the result of the rollback
*/
public final ICommandResult rollback(T provider) {
return rollback(provider, true);
}
/**
* This method launch the rollback of the command execution (or chain of commands) using the default
* default Executor.
* The rollback is effectively performed only if the command has been executed with a positive result, otherwise
* the command is intended as "not executed" then no rollback will be performed.
* This command is the same of:
* rollback(provider, new DefaultCommandExecutor(), catchRuntimeException);
* @param catchRuntimeException whether catch or propagate a RuntimeException
* @return the result of the rollback
*/
public final ICommandResult rollback(T provider, boolean catchRuntimeException) {
return rollback(provider, new DefaultCommandExecutor(), catchRuntimeException);
}
/**
* This method launch the rollback of the command execution (or chain of commands) using a custom command executor.
* The rollback is effectively performed only if the command has been executed with a positive result, otherwise
* the command is intended as "not executed" then no rollback will be performed.
* This command is the same of:
* rollback(provider, commandExecutor, true);
* @return the result of the rollback
*/
public final ICommandResult rollback(T provider, ICommandExecutor commandExecutor) {
return rollback(provider, commandExecutor, true);
}
/**
* This method launch the rollback of the command execution (or chain of commands) using a custom command executor.
* The rollback is effectively performed only if the command has been executed with a positive result, otherwise
* the command is intended as "not executed" then no rollback will be performed.
* If the catchRuntimeException is true every RuntimeException will be caught and a new ErrorMessage in the
* error list is created; otherwise the Runtime Exception will be propagated to the command caller.
* @return the result of the rollback
*/
public final ICommandResult rollback(T provider, ICommandExecutor commandExecutor, boolean catchRuntimeException) {
visit(provider);
return rollback(commandExecutor, catchRuntimeException, new CommandResult());
}
void visit(T provider) {
this.provider = provider;
}
protected final ICommandResult exec(ICommandExecutor commandExecutor, boolean catchRuntimeException, ACommandResult commandResult) {
this.commandExecutor = commandExecutor;
getCommandExecutor().execute(this, catchRuntimeException, commandResult);
return commandResult;
}
protected final ICommandResult rollback(ICommandExecutor commandExecutor, boolean catchRuntimeException, ACommandResult commandResult) {
this.commandExecutor = commandExecutor;
getCommandExecutor().rollback(this, catchRuntimeException ,commandResult);
return commandResult;
}
protected final ICommandExecutor getCommandExecutor() {
if (commandExecutor==null) {
commandExecutor = new DefaultCommandExecutor();
}
return commandExecutor;
}
protected final T getProvider() {
if (provider==null) {
throw new NullProviderException();
}
return provider;
}
protected final ILogger getLogger() {
if (logger == null) {
if (provider == null) {
logger = new SystemOutLoggerFactory().logger(getClass());
} else {
logger = getProvider().getLoggerService().logger(this.getClass());
}
}
return logger;
}
protected abstract void doExec(boolean catchRuntimeException, ACommandResult commandResult);
protected abstract void doRollback(boolean catchRuntimeException, ACommandResult commandResult);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy