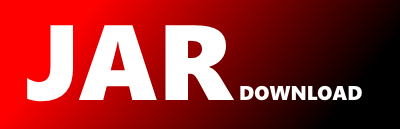
com.jpattern.service.cache.ehcache.EhCache Maven / Gradle / Ivy
package com.jpattern.service.cache.ehcache;
import java.io.Serializable;
import com.jpattern.service.cache.ACache;
import com.jpattern.service.cache.statistics.ICacheStatistics;
import net.sf.ehcache.CacheManager;
import net.sf.ehcache.Ehcache;
import net.sf.ehcache.Element;
/**
*
* @author Francesco Cina'
*
* 2 May 2011
*/
public class EhCache extends ACache {
private Ehcache ehcache;
public EhCache(String name, CacheManager cacheManager, ICacheStatistics cacheStatistics) {
super(name, cacheStatistics);
ehcache = cacheManager.getEhcache(name);
}
@Override
public synchronized Serializable getValue(String key) {
if (ehcache!=null) {
Element element = ehcache.get(key);
if (element != null) {
return element.getValue();
}
}
return null;
}
@Override
public synchronized void put(String key, Serializable value) {
if (ehcache!=null) {
ehcache.put(new Element(key, value));
}
}
@Override
public synchronized void clear() {
if (ehcache!=null) {
ehcache.removeAll();
}
}
@Override
public synchronized void remove(String key) {
if (ehcache!=null) {
ehcache.remove(key);
}
}
@Override
public boolean contains(String key) {
return ( (ehcache!=null) && (ehcache.get(key)!=null) );
}
@Override
public int getSize() {
return ehcache.getSize();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy