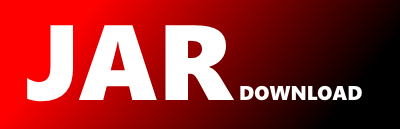
com.jpattern.orm.generator.ResultSetMethodHelper Maven / Gradle / Ivy
package com.jpattern.orm.generator;
import java.io.InputStream;
import java.io.Reader;
import java.lang.reflect.Method;
import java.math.BigDecimal;
import java.net.URL;
import java.sql.Array;
import java.sql.Blob;
import java.sql.Clob;
import java.sql.Date;
import java.sql.NClob;
import java.sql.Ref;
import java.sql.ResultSet;
import java.sql.RowId;
import java.sql.SQLXML;
import java.sql.Time;
import java.sql.Timestamp;
import java.util.Map;
import java.util.HashMap;
import com.jpattern.orm.exception.OrmConfigurationException;
import com.jpattern.orm.generator.wrapper.IWrapper;
import com.jpattern.orm.generator.wrapper.JodaDateTimeToSqlTimestampWrapper;
import com.jpattern.orm.generator.wrapper.JodaLocalDateTimeToSqlTimestampWrapper;
import com.jpattern.orm.generator.wrapper.JodaLocalDateToSqlTimestampWrapper;
import com.jpattern.orm.generator.wrapper.UtilDateToSqlTimestampWrapper;
/**
*
* @author Francesco Cina
*
* 06/giu/2011
*/
public abstract class ResultSetMethodHelper {
private static boolean INITIALISED = false;
private static Map, Method> RESULTSET_GETTER_FOR_INT = new HashMap, Method>();
private static Map, Method> RESULTSET_SETTER_FOR_STRING = new HashMap, Method>();
private static Map, IWrapper, ?>> WRAPPER_METHODS = new HashMap, IWrapper, ?>>();
public static final Method findResultSetGetterForString(final Class> type) throws SecurityException, NoSuchMethodException, OrmConfigurationException {
init();
if (RESULTSET_SETTER_FOR_STRING.containsKey(type)) {
return RESULTSET_SETTER_FOR_STRING.get(type);
}
throw new OrmConfigurationException("Type [" + type + "] is not supported at this time");
}
public static final Method findResultSetGetterForInt(final Class> type) throws SecurityException, NoSuchMethodException, OrmConfigurationException {
init();
if (RESULTSET_GETTER_FOR_INT.containsKey(type)) {
return RESULTSET_GETTER_FOR_INT.get(type);
}
throw new OrmConfigurationException("Type [" + type + "] is not supported at this time");
}
private static void init() throws SecurityException, NoSuchMethodException {
if (!INITIALISED) {
addGetter(Array.class, "getArray");
addGetter(BigDecimal.class, "getBigDecimal");
addGetter(InputStream.class, "getBinaryStream");
addGetter(Blob.class, "getBlob");
addGetter(Boolean.TYPE, "getBoolean");
addGetter(Boolean.class, "getBoolean");
addGetter(Byte.TYPE, "getByte");
addGetter(Byte.class, "getByte");
addGetter(byte[].class, "getBytes");
addGetter(Reader.class, "getCharacterStream");
addGetter(Clob.class, "getClob");
addGetter(Date.class, "getDate");
addGetter(Double.TYPE, "getDouble");
addGetter(Double.class, "getDouble");
addGetter(Float.TYPE, "getFloat");
addGetter(Float.class, "getFloat");
addGetter(Integer.TYPE, "getInt");
addGetter(Integer.class, "getInt");
addGetter(Long.TYPE, "getLong");
addGetter(Long.class, "getLong");
addGetter(NClob.class, "getNClob");
addGetter(Object.class, "getObject");
addGetter(Ref.class, "getRef");
addGetter(RowId.class, "getRowId");
addGetter(Short.TYPE, "getShort");
addGetter(Short.class, "getShort");
addGetter(SQLXML.class, "getSQLXML");
addGetter(String.class, "getString");
addGetter(Time.class, "getTime");
addGetter(Timestamp.class, "getTimestamp");
addGetter(URL.class, "getURL");
INITIALISED = true;
//After initialization
addWrappers(java.util.Date.class , new UtilDateToSqlTimestampWrapper());
try {
addWrappers(org.joda.time.DateTime.class , new JodaDateTimeToSqlTimestampWrapper());
addWrappers(org.joda.time.LocalDateTime.class , new JodaLocalDateTimeToSqlTimestampWrapper());
addWrappers(org.joda.time.LocalDate.class , new JodaLocalDateToSqlTimestampWrapper());
} catch (Throwable e) {}
}
}
private static void addGetter(Class> key, String resultSetMethodName) throws SecurityException, NoSuchMethodException {
RESULTSET_GETTER_FOR_INT.put(key, ResultSet.class.getMethod(resultSetMethodName, new Class[] { int.class }));
RESULTSET_SETTER_FOR_STRING.put(key, ResultSet.class.getMethod(resultSetMethodName, new Class[] { String.class }));
}
private static void addWrappers(Class clazz, IWrapper wrapper) throws SecurityException, OrmConfigurationException, NoSuchMethodException {
WRAPPER_METHODS.put(clazz, wrapper);
addGetter( clazz , findResultSetGetterForInt( wrapper.resultSetTypeToUse() ).getName() );
}
@SuppressWarnings("unchecked")
public static IWrapper getWrapper(Class clazz) throws SecurityException, NoSuchMethodException {
init();
if (WRAPPER_METHODS.containsKey(clazz)) {
return (IWrapper) WRAPPER_METHODS.get(clazz);
}
throw new OrmConfigurationException("Type [" + clazz + "] has no wrapper associated");
}
public static boolean isWrappedType(Class> clazz) throws SecurityException, NoSuchMethodException {
init();
return WRAPPER_METHODS.containsKey(clazz);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy