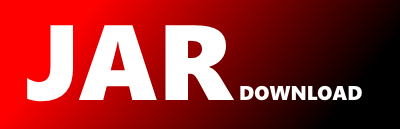
com.jpattern.orm.query.clause.From Maven / Gradle / Ivy
package com.jpattern.orm.query.clause;
import com.jpattern.orm.query.INameSolverConsumer;
import com.jpattern.orm.query.IQueryRoot;
import com.jpattern.orm.query.IRenderableSqlObject;
/**
*
* @author Francesco Cina
*
* 18/giu/2011
*/
public interface From extends SqlClauseRoot, INameSolverConsumer, IRenderableSqlObject {
/**
* Perform a simple (cross) Join.
* This join returns the Cartesian product of rows from tables in the join.
* A cross join is the join commonly used when more tables are comma
* separated in a from clause.
* The name of the class will be used as alias.
* @return
*/
From join(Class> joinClass);
/**
* Perform a simple (cross) Join.
* This join returns the Cartesian product of rows from tables in the join.
* A cross join is the join commonly used when more tables are comma
* separated in a from clause.
* @return
*/
From join(Class> joinClass, String joinClassAlias);
/**
* Perform a natural Join.
* The join predicate arises implicitly by comparing all columns in both tables that have the same column-names in the joined tables. The resulting joined table contains only one column for each pair of equally-named columns.
* The name of the class will be used as alias.
* @return
*/
From naturalJoin(Class> joinClass);
/**
* Perform a natural Join.
* The join predicate arises implicitly by comparing all columns in both tables that have the same column-names in the joined tables. The resulting joined table contains only one column for each pair of equally-named columns..
* @return
*/
From naturalJoin(Class> joinClass, String joinClassAlias);
/**
* Perform a inner Join.
* An inner join can be performed in a normal sql query simply using the key JOIN.
* @return
*/
From innerJoin(Class> joinClass);
/**
* Perform a inner Join.
* An inner join can be performed in a normal sql simply using the key JOIN.
* @return
*/
From innerJoin(Class> joinClass, String joinClassAlias);
/**
* Perform a inner Join.
* An inner join can be performed in a normal sql query simply using the key JOIN.
* @return
*/
From innerJoin(Class> joinClass, String onLeftProperty, String onRigthProperty);
/**
* Perform a inner Join.
* An inner join can be performed in a normal sql simply using the key JOIN.
* @return
*/
From innerJoin(Class> joinClass, String joinClassAlias, String onLeftProperty, String onRigthProperty);
/**
* Perform a natural left outer Join.
* The name of the class will be used as alias.
* @return
*/
From leftOuterJoin(Class> joinClass);
/**
* Perform a natural left outer Join.
* @return
*/
From leftOuterJoin(Class> joinClass, String joinClassAlias);
/**
* Perform a left outer Join.
* @return
*/
From leftOuterJoin(Class> joinClass, String onLeftProperty, String onRigthProperty);
/**
* Perform left outer Join.
* @return
*/
From leftOuterJoin(Class> joinClass, String joinClassAlias, String onLeftProperty, String onRigthProperty);
/**
* Perform a natural right outer Join.
* The name of the class will be used as alias.
* @return
*/
From rightOuterJoin(Class> joinClass);
/**
* Perform a natural right outer Join.
* @return
*/
From rightOuterJoin(Class> joinClass, String joinClassAlias);
/**
* Perform a right outer Join.
* @return
*/
From rightOuterJoin(Class> joinClass, String onLeftProperty, String onRigthProperty);
/**
* Perform right outer Join.
* @return
*/
From rightOuterJoin(Class> joinClass, String joinClassAlias, String onLeftProperty, String onRigthProperty);
/**
* Perform a natural full outer Join.
* The name of the class will be used as alias.
* @return
*/
From fullOuterJoin(Class> joinClass);
/**
* Perform a natural full outer Join.
* @return
*/
From fullOuterJoin(Class> joinClass, String joinClassAlias);
/**
* Perform a full outer Join.
* @return
*/
From fullOuterJoin(Class> joinClass, String onLeftProperty, String onRigthProperty);
/**
* Perform full outer Join.
* @return
*/
From fullOuterJoin(Class> joinClass, String joinClassAlias, String onLeftProperty, String onRigthProperty);
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy