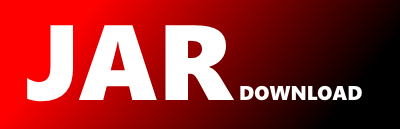
com.jpattern.orm.query.clause.Where Maven / Gradle / Ivy
package com.jpattern.orm.query.clause;
import java.util.Collection;
import java.util.Map;
import com.jpattern.orm.query.BaseFindQuery;
import com.jpattern.orm.query.IQueryRoot;
import com.jpattern.orm.query.where.ExpressionElement;
/**
*
* @author Francesco Cina
*
* 18/giu/2011
*/
public interface Where extends SqlClauseRoot, ExpressionElement {
/**
* All Equal - Map containing property names and their values.
* @param propertyMap
* @return
*/
Where allEq(Map propertyMap);
/**
* Express the "Equals to" relation between an object's property
* and a fixed value.
*
* @param property
* @param value
* @return
*/
Where eq(String property, Object value);
/**
* Express the "Equals to" relation between objects properties
*
* @param firstProperty
* @param secondProperty
* @return
*/
Where eqProperties(String firstProperty, String secondProperty);
/**
* Express the "Lesser or equals to" relation between an object's property
* and a fixed value.
*
* @param property
* @param value
* @return
*/
Where le(String property, Object value);
/**
* Express the "Lesser or equals to" relation between objects properties
*
* @param firstProperty
* @param secondProperty
* @return
*/
Where leProperties(String firstProperty, String secondProperty);
/**
* Express the "Greater or equals to" relation between an object's property
* and a fixed value.
*
* @param property
* @param value
* @return
*/
Where ge(String property, Object value);
/**
* Express the "Greater or equals to" relation between objects properties
*
* @param firstProperty
* @param secondProperty
* @return
*/
Where geProperties(String firstProperty, String secondProperty);
/**
*
* Express the "Lesser than" relation between an object's property
* and a fixed value.
*
* @param property
* @param value
* @return
*/
Where lt(String property, Object value);
/**
* Express the "Lesser than" relation between objects properties
*
* @param firstProperty
* @param secondProperty
* @return
*/
Where ltProperties(String firstProperty, String secondProperty);
/**
* Express the "Greater than" relation between an object's property
* and a fixed value.
*
* @param property
* @param value
* @return
*/
Where gt(String property, Object value);
/**
* Express the "Greater than" relation between objects properties
*
* @param firstProperty
* @param secondProperty
* @return
*/
Where gtProperties(String firstProperty, String secondProperty);
/**
* Express the "Insensitive Equal To" between an object's property
* and a fixed value (it uses a lower() function to make both case insensitive).
*
* @param propertyName
* @param value
* @return
*/
Where ieq(String property, String value);
/**
* Express the "Insensitive Equal To" bbetween objects properties
* (it uses a lower() function to make both case insensitive).
*
* @param firstProperty
* @param secondProperty
* @return
*/
Where ieqProperties(String firstProperty, String secondProperty);
/**
* Express the "Not Equals to" relation between objects properties.
*
* @param property
* @param value
* @return
*/
Where ne(String property, Object value);
/**
* Express the "Not Equals to" relation between an object's property
* and a fixed value.
*
* @param firstProperty
* @param secondProperty
* @return
*/
Where neProperties(String firstProperty, String secondProperty);
/**
* Case insensitive Like - property like value where the value contains the
* SQL wild card characters % (percentage) and _ (underscore).
*
* @param propertyName
* @param value
* @return
*/
Where ilike(String property, String value);
/**
* Not In - property has a value in the collection of values.
*
* @param propertyName
* @param values
* @return
*/
Where nin(String property, Collection> values);
/**
* Not In - property has a value in the array of values.
*
* @param propertyName
* @param values
* @return
*/
Where nin(String property, Object[] values);
/**
* Not In - using a subQuery.
*
* @param propertyName
* @param subQuery
* @return
*/
Where nin(String property, BaseFindQuery subQuery);
/**
* In - property has a value in the collection of values.
*
* @param propertyName
* @param values
* @return
*/
Where in(String property, Collection> values);
/**
* In - property has a value in the array of values.
*
* @param propertyName
* @param values
* @return
*/
Where in(String property, Object[] values);
/**
* In - using a subQuery.
*
* @param propertyName
* @param subQuery
* @return
*/
Where in(String property, BaseFindQuery subQuery);
/**
* Is Not Null - property is not null.
*
* @param propertyName
* @return
*/
Where isNotNull(String property);
/**
* Is Null - property is null.
*
* @param propertyName
* @return
*/
Where isNull(String property);
/**
* Like - property like value where the value contains the SQL wild card
* characters % (percentage) and _ (underscore).
*
* @param propertyName
* @param value
*/
Where like(String property, String value);
/**
* Not Like - property like value where the value contains the SQL wild card
* characters % (percentage) and _ (underscore).
*
* @param propertyName
* @param value
*/
Where nlike(String property, String value);
/**
* Negate the expression (prefix it with NOT).
*
* @param exp
* @return
*/
Where not(ExpressionElement expression);
/**
* Or - join two expressions with a logical or.
*
* @param expOne
* @param expTwo
* @return
*/
Where or(ExpressionElement expressionOne, ExpressionElement expressionTwo);
/**
* And - join two expressions with a logical and.
*
* @param expOne
* @param expTwo
* @return
*/
Where and(ExpressionElement expressionOne, ExpressionElement expressionTwo);
/**
* Return the current query object
* @return
*/
@Override
T query();
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy