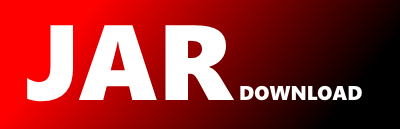
com.jpattern.orm.session.Session Maven / Gradle / Ivy
package com.jpattern.orm.session;
import java.util.List;
import com.jpattern.orm.exception.OrmException;
import com.jpattern.orm.exception.OrmNotUniqueResultException;
import com.jpattern.orm.query.DeleteQuery;
import com.jpattern.orm.query.CustomFindQuery;
import com.jpattern.orm.query.FindQuery;
import com.jpattern.orm.query.SqlExecutor;
import com.jpattern.orm.query.UpdateQuery;
import com.jpattern.orm.script.IScriptExecutor;
import com.jpattern.orm.transaction.ITransaction;
import com.jpattern.orm.transaction.ITransactionDefinition;
/**
*
* @author Francesco Cina
*
* 21/mag/2011
*
*/
public interface Session {
/**
* Begin a transaction or participate to an existing one using the default ITransactionDefinition
* @return
* @throws OrmException
*/
ITransaction transaction() throws OrmException;
/**
* Begin a or participate to an existing one depending on the specific transactionDefinition
* @return
* @throws OrmException
*/
ITransaction transaction(ITransactionDefinition transactionDefinition) throws OrmException;
/**
* Find a bean using is ID.
* @param
* @param clazz The Class of the object to load
* @param idValue the value of the identifying column of the object
* @return
* @throws OrmException
*/
T find(Class clazz, Object idValue) throws OrmException;
/**
* Find a bean using is IDs.
* @param
* @param clazz The Class of the object to load
* @param idValues an ordered array with the values of the identifying columns of the object
* @return
* @throws OrmException
*/
T find(Class clazz, Object[] idValues) throws OrmException;
/**
* Find a bean using is ID. An OrmNotUniqueResultException is thrown if nothing is found.
* @param
* @param clazz The Class of the object to load
* @param idValue the value of the identifying column of the object
* @return
* @throws OrmException
*/
T findUnique(Class clazz, Object idValue) throws OrmException, OrmNotUniqueResultException;
/**
* Find a bean using is IDs. An OrmNotUniqueResultException is thrown if nothing is found.
* @param
* @param clazz The Class of the object to load
* @param idValues an ordered array with the values of the identifying columns of the object
* @return
* @throws OrmException
*/
T findUnique(Class clazz, Object[] idValues) throws OrmException, OrmNotUniqueResultException;
/**
* Persist the new object in the database
* @param
* @param object
* @throws OrmException
*/
void save(T object) throws OrmException;
/**
* Persist the new objects in the database
* @param
* @param objects the objects to persist
* @throws OrmException
*/
void save(List objects) throws OrmException;
/**
* Update the values of an existing object in the database
* @param
* @param object
* @throws OrmException
*/
void update(T object) throws OrmException;
/**
* Update the values of the existing objects in the database
* @param
* @param objects the objects to update
* @throws OrmException
*/
void update(List objects) throws OrmException;
/**
* Update the objects of a specific TABLE
* @param clazz the TABLE related Class
* @throws OrmException
*/
UpdateQuery updateQuery(Class> clazz) throws OrmException;
/**
* Update the objects of a specific TABLE
* @param clazz the TABLE related Class
* @param alias The alias of the class in the query.
* @throws OrmException
*/
UpdateQuery updateQuery(Class> clazz, String alias) throws OrmException;
/**
* Delete one object from the database
* @param
* @param object
* @throws OrmException
*/
void delete(T object) throws OrmException;
/**
* Delete the objects from the database
* @param
* @param objects the objects to delete
* @throws OrmException
*/
void delete(List object) throws OrmException;
/**
* Delete the objects of a specific table
* @param clazz the TABLE related Class
* @throws OrmException
*/
DeleteQuery deleteQuery(Class> clazz) throws OrmException;
/**
* Delete the objects of a specific table
* @param clazz the TABLE related Class
* @param alias The alias of the class in the query.
* @throws OrmException
*/
DeleteQuery deleteQuery(Class> clazz, String alias) throws OrmException;
/**
* Create a new query
* @param
* @param clazz The class of the object that will be retrieved by the query execution. The simple class name will be used as alias for the class
* @return
* @throws OrmException
*/
FindQuery findQuery(Class clazz ) throws OrmException;
/**
* Create a new query
* @param
* @param clazz The class of the object that will be retrieved by the query execution.
* @param alias The alias of the class in the query.
* @return
* @throws OrmException
*/
FindQuery findQuery(Class clazz, String alias ) throws OrmException;
/**
* Create a new query
* @param selectClause the select clause of the query (do not use the "select" keyword)
* @param clazz The class of the object that will be retrieved by the query execution.
* @param alias The alias of the class in the query.
* @return
* @throws OrmException
*/
CustomFindQuery findQuery(String selectClause, Class> clazz, String alias ) throws OrmException;
/**
* An executor to perform any kind of plain SQL statements.
* @return
*/
SqlExecutor sqlExecutor();
/**
* A script executor useful to execute multiple sql statement from files.
* @return
* @throws OrmException
*/
IScriptExecutor scriptExecutor() throws OrmException;
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy