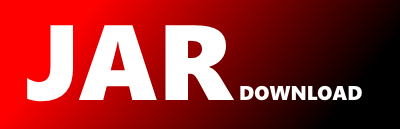
com.jpattern.orm.transaction.TransactionDefinition Maven / Gradle / Ivy
package com.jpattern.orm.transaction;
/**
* Definition of a new Transaction.
* Default values are:
*
* Transaction propagation: REQUIRED
* Isolation level: the default of the actual jdbc driver
* Timeout: the default of the actual jdbc driver
* ReadOnly: false
*
* @author cinafr
*
*/
public class TransactionDefinition implements ITransactionDefinition {
private static final long serialVersionUID = 1L;
private final TransactionPropagation propagation;
private final TransactionIsolation isolationLevel;
private int timeout;
private final boolean readOnly;
public TransactionDefinition() {
this(PROPAGATION_DEFAULT, ISOLATION_DEFAULT, READ_ONLY_DEFAULT, TIMEOUT_DEFAULT);
}
public TransactionDefinition(TransactionPropagation propagation) {
this(propagation, ISOLATION_DEFAULT, READ_ONLY_DEFAULT, TIMEOUT_DEFAULT);
}
public TransactionDefinition(TransactionIsolation isolationLevel) {
this(PROPAGATION_DEFAULT, isolationLevel, READ_ONLY_DEFAULT, TIMEOUT_DEFAULT);
}
public TransactionDefinition(boolean readOnly) {
this(PROPAGATION_DEFAULT, ISOLATION_DEFAULT, readOnly, TIMEOUT_DEFAULT);
}
public TransactionDefinition(TransactionPropagation propagation, TransactionIsolation isolationLevel) {
this(propagation, isolationLevel, READ_ONLY_DEFAULT, TIMEOUT_DEFAULT);
}
public TransactionDefinition(TransactionIsolation isolationLevel, boolean readOnly) {
this(PROPAGATION_DEFAULT, isolationLevel, readOnly, TIMEOUT_DEFAULT);
}
public TransactionDefinition(TransactionPropagation propagation, boolean readOnly) {
this(propagation, ISOLATION_DEFAULT, readOnly, TIMEOUT_DEFAULT);
}
public TransactionDefinition(TransactionPropagation propagation, TransactionIsolation isolationLevel, boolean readOnly) {
this(propagation, isolationLevel, readOnly, TIMEOUT_DEFAULT);
}
public TransactionDefinition(TransactionPropagation propagation, TransactionIsolation isolationLevel, boolean readOnly, int timeout) {
this.propagation = propagation;
this.isolationLevel = isolationLevel;
this.setTimeout(timeout);
this.readOnly = readOnly;
}
@Override
public TransactionPropagation getPropagation() {
return propagation;
}
@Override
public TransactionIsolation getIsolationLevel() {
return isolationLevel;
}
@Override
public int getTimeout() {
return timeout;
}
public void setTimeout(int timeout) {
this.timeout = timeout;
}
@Override
public boolean isReadOnly() {
return readOnly;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy