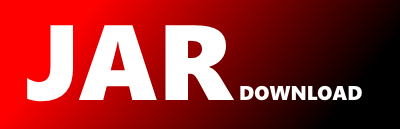
com.jpattern.orm.test.AutoIdTest Maven / Gradle / Ivy
The newest version!
package com.jpattern.orm.test;
import static org.junit.Assert.assertEquals;
import static org.junit.Assert.assertNotNull;
import static org.junit.Assert.assertNull;
import static org.junit.Assert.assertTrue;
import java.util.Date;
import org.junit.Test;
import com.jpattern.orm.BaseTestShared;
import com.jpattern.orm.JPO;
import com.jpattern.orm.session.Session;
import com.jpattern.orm.test.domain.AutoId;
import com.jpattern.orm.test.domain.AutoIdInteger;
import com.jpattern.orm.transaction.Transaction;
/**
*
* @author Francesco Cina
*
* 20/mag/2011
*/
public class AutoIdTest extends BaseTestShared {
@Override
protected void setUp() throws Exception {
}
@Override
protected void tearDown() throws Exception {
}
@Test
public void testAutoId() throws Exception {
final JPO jpOrm = getJPOrm();
jpOrm.register(AutoId.class);
AutoId autoId = new AutoId();
autoId.setValue("value for test " + new Date().getTime() );
// CREATE
final Session conn = jpOrm.session();
Transaction tx = conn.transaction();
autoId = conn.save(autoId);
tx.commit();
System.out.println("autoId id: " + autoId.getId());
assertTrue( autoId.getId() >= 100 );
// LOAD
AutoId autoIdLoad1 = conn.find(AutoId.class, autoId.getId() );
assertNotNull(autoIdLoad1);
assertEquals( autoId.getId(), autoIdLoad1.getId() );
assertEquals( autoId.getValue(), autoIdLoad1.getValue() );
//UPDATE
tx = conn.transaction();
autoIdLoad1.setValue("new Value " + new Date().getTime() );
autoIdLoad1 = conn.update(autoIdLoad1);
tx.commit();
// LOAD
final AutoId autoIdLoad2 = conn.find(AutoId.class, autoId.getId() );
assertNotNull(autoIdLoad2);
assertEquals( autoIdLoad1.getId(), autoIdLoad2.getId() );
assertEquals( autoIdLoad1.getValue(), autoIdLoad2.getValue() );
//DELETE
tx = conn.transaction();
conn.delete(autoIdLoad2);
tx.commit();
final AutoId autoIdLoad3 = conn.find(AutoId.class, autoId.getId() );
assertNull(autoIdLoad3);
}
@Test
public void testAutoIdInteger() throws Exception {
final JPO jpOrm = getJPOrm();
jpOrm.register(AutoIdInteger.class);
AutoIdInteger autoId = new AutoIdInteger();
autoId.setValue("value for test " + new Date().getTime() );
// CREATE
final Session conn = jpOrm.session();
Transaction tx = conn.transaction();
autoId = conn.save(autoId);
tx.commit();
System.out.println("autoId id: " + autoId.getId());
assertTrue( autoId.getId() >= 100 );
// LOAD
AutoIdInteger autoIdLoad1 = conn.find(AutoIdInteger.class, autoId.getId() );
assertNotNull(autoIdLoad1);
assertEquals( autoId.getId(), autoIdLoad1.getId() );
assertEquals( autoId.getValue(), autoIdLoad1.getValue() );
//UPDATE
tx = conn.transaction();
autoIdLoad1.setValue("new Value " + new Date().getTime() );
autoIdLoad1 = conn.update(autoIdLoad1);
tx.commit();
// LOAD
final AutoIdInteger autoIdLoad2 = conn.find(AutoIdInteger.class, autoId.getId() );
assertNotNull(autoIdLoad2);
assertEquals( autoIdLoad1.getId(), autoIdLoad2.getId() );
assertEquals( autoIdLoad1.getValue(), autoIdLoad2.getValue() );
//DELETE
tx = conn.transaction();
conn.delete(autoIdLoad2);
tx.commit();
final AutoIdInteger autoIdLoad3 = conn.find(AutoIdInteger.class, autoId.getId() );
assertNull(autoIdLoad3);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy