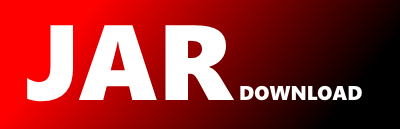
com.jpattern.orm.test.transaction.TransactionCallbackTest Maven / Gradle / Ivy
The newest version!
package com.jpattern.orm.test.transaction;
import static org.junit.Assert.assertNotNull;
import static org.junit.Assert.assertNull;
import static org.junit.Assert.assertTrue;
import static org.junit.Assert.fail;
import java.util.ArrayList;
import java.util.List;
import java.util.Random;
import org.junit.Test;
import com.jpattern.orm.BaseTestShared;
import com.jpattern.orm.exception.OrmException;
import com.jpattern.orm.session.Session;
import com.jpattern.orm.session.TransactionCallback;
import com.jpattern.orm.test.domain.Employee;
import com.jpattern.orm.transaction.Transaction;
/**
*
* @author Francesco Cina
*
* 20/mag/2011
*/
public class TransactionCallbackTest extends BaseTestShared {
private final int repeatTests = 50;
private Session session;
@Override
protected void setUp() throws Exception {
this.session = getJPOrm().session();
}
@Override
protected void tearDown() throws Exception {
}
@Test
public void testTransactionCommitted() throws Exception {
final Random random = new Random();
final List employees = new ArrayList();
for (int i=0 ; i() {
@Override
public Void doInTransaction(final Session session) {
final Employee employee = new Employee();
employee.setId( random.nextInt() );
employees.add(employee);
session.save(employee);
return null;
}
});
}
for (Employee employee : employees) {
assertNotNull( this.session.find(Employee.class, employee.getId()) );
}
}
@Test
public void testTransactionRolledback() throws Exception {
final Random random = new Random();
final List employees = new ArrayList();
for (int i=0 ; i() {
@Override
public Void doInTransaction(final Session session) {
final Employee employee = new Employee();
employee.setId( random.nextInt() );
employees.add(employee);
session.save(employee);
throw new RuntimeException("manually thrown exception");
}
});
}
catch (RuntimeException e) {
//nothing to do
}
}
for (Employee employee : employees) {
assertNull( this.session.find(Employee.class, employee.getId()) );
}
}
@Test
public void testPartecipatingInExistingTransaction() throws Exception {
final Random random = new Random();
final List employees = new ArrayList();
Transaction tx = this.session.transaction();
for (int i=0 ; i() {
@Override
public Void doInTransaction(final Session session) {
final Employee employee = new Employee();
employee.setId( random.nextInt() );
employees.add(employee);
session.save(employee);
return null;
}
});
}
tx.rollback();
for (Employee employee : employees) {
assertNull( this.session.find(Employee.class, employee.getId()) );
}
}
@Test
public void testPartecipatingInExistingTransactionAndRollback() throws Exception {
final Random random = new Random();
final List employees = new ArrayList();
Transaction tx = this.session.transaction();
for (int i=0 ; i() {
@Override
public Void doInTransaction(final Session session) {
final Employee employee = new Employee();
employee.setId( random.nextInt() );
employees.add(employee);
session.save(employee);
return null;
}
});
}
try {
this.session.doInTransaction(new TransactionCallback() {
@Override
public Void doInTransaction(final Session session) {
final Employee employee = new Employee();
employee.setId( random.nextInt() );
employees.add(employee);
session.save(employee);
throw new RuntimeException("manually thrown exception");
}
});
}
catch (RuntimeException e) {
//nothing to do
}
try {
tx.commit();
fail();
} catch (OrmException e) {
assertTrue(e.getMessage().contains("rollback"));
}
for (Employee employee : employees) {
assertNull( this.session.find(Employee.class, employee.getId()) );
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy