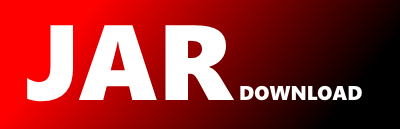
com.jpattern.orm.query.CustomQuery Maven / Gradle / Ivy
package com.jpattern.orm.query;
import java.math.BigDecimal;
import java.util.List;
import com.jpattern.orm.exception.OrmException;
import com.jpattern.orm.exception.OrmNotUniqueResultException;
import com.jpattern.orm.session.ResultSetReader;
/**
*
* @author Francesco Cina
*
* 09/lug/2011
*/
public interface CustomQuery {
/**
* Execute the query reading the ResultSet with a
* IResultSetReader.
* @param rse object that will extract all rows of results
* @return an arbitrary result object, as returned by the IResultSetExtractor
*/
T find(ResultSetReader rse) throws OrmException;
/**
* Execute the query and read the result creating a List of all the ordered arrays with the extracted column values for every row.
* @return
*/
List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy