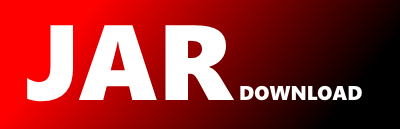
com.jpattern.orm.query.find.FindQueryCommon Maven / Gradle / Ivy
package com.jpattern.orm.query.find;
import java.util.List;
import com.jpattern.orm.exception.OrmException;
import com.jpattern.orm.exception.OrmNotUniqueResultException;
import com.jpattern.orm.query.BaseFindQuery;
import com.jpattern.orm.query.LockMode;
import com.jpattern.orm.query.OrmRowMapper;
/**
*
* @author Francesco Cina
*
* 18/giu/2011
*/
public interface FindQueryCommon extends BaseFindQuery {
/**
* Execute the query returning the list of objects.
* @return
*/
List findList() throws OrmException;
/**
* Return the count of entities this query should return.
* @return
*/
long findRowCount() throws OrmException;
/**
* Execute the query returning either a single bean or null (if no matching bean is found).
* @return
* @throws OrmNotUniqueResultException if zero or more than one row are returned from the query
*/
BEAN findUnique() throws OrmException, OrmNotUniqueResultException;
/**
* Execute the query and for every object created call the IOrmSerialResultReader.
* The objects are created one after the other to avoid the use of too much memory.
* @param srr
* @throws OrmException
*/
void find(OrmRowMapper srr) throws OrmException;
/**
* Return the sql that was generated to return the row count of the execution of this query.
* @return
*/
String getGeneratedRowCountSql() throws OrmException;
/**
* Whether to use Distinct in the select clause
* @return
*/
FindQuery setDistinct(boolean distinct) throws OrmException;
/**
* return if use Distinct in the select clause
* @return
*/
boolean isDistinct() throws OrmException;
/**
*
* @param lockMode
* @return
*/
FindQuery setLockMode(LockMode lockMode);
/**
* Return the current lock mode
* @return
*/
LockMode getLockMode();
/**
* Return the max rows for this query.
* @return
*/
int getMaxRows() throws OrmException;
/**
* Set the maximum number of rows to return in the query.
* @param maxRows
* @return
*/
FindQuery setMaxRows(int maxRows) throws OrmException;
/**
* Set the query timeout for the query.
*/
FindQuery setQueryTimeout(int queryTimeout);
/**
* Return the query timeout for the query.
*/
int getQueryTimeout();
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy