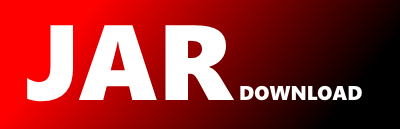
com.jpattern.orm.query.sql.SqlExecutor Maven / Gradle / Ivy
package com.jpattern.orm.query.sql;
import java.util.List;
import com.jpattern.orm.exception.OrmException;
import com.jpattern.orm.session.GeneratedKeyReader;
import com.jpattern.orm.session.BatchPreparedStatementSetter;
/**
*
* @author Francesco Cina
*
* 09/lug/2011
*/
public interface SqlExecutor {
/**
* Perform a single SQL select operation.
* @param sql static SQL to execute
* @param args arguments to bind to the query
* @return
*/
SqlFindQuery findQuery(String sql, Object... args);
/**
* Issue a single SQL execute, typically a DDL statement.
* @param sql static SQL to execute
*/
SqlExecuteQuery executeQuery(String sql) throws OrmException;
/**
* Perform a single SQL update operation (such as an insert, update or delete statement).
* @param sql static SQL to execute
* @param args arguments to bind to the query
* @return
*/
SqlUpdateQuery updateQuery(String sql, Object... args) throws OrmException;
/**
* Issue an update statement using a PreparedStatementCreator to provide SQL and
* any required parameters. Generated keys can be read using the IGeneratedKeyReader.
* @param psc object that provides SQL and any necessary parameters
* @param generatedKeyReader IGeneratedKeyReader to read the generated key
* @return
*/
SqlUpdateQuery updateQuery(String sql, GeneratedKeyReader generatedKeyReader, Object... args) throws OrmException;
/**
* Issue multiple SQL updates on a single JDBC Statement using batching.
* @param sql defining a List of SQL statements that will be executed.
* @return
*/
SqlBatchUpdateQuery batchUpdate(List sqls) throws OrmException;
/**
* Issue multiple SQL updates on a single JDBC Statement using batching.
* The same query is executed for every Object array present in the args list
* which is the list of arguments to bind to the query.
* @param sql defining a List of SQL statements that will be executed.
* @param args defining a List of Object arrays to bind to the query.
* @return
*/
SqlBatchUpdateQuery batchUpdate(String sql, List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy