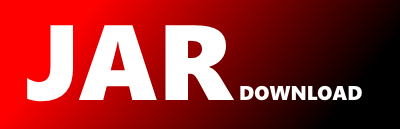
com.jpattern.orm.session.SessionProvider Maven / Gradle / Ivy
package com.jpattern.orm.session;
import java.sql.Connection;
import java.sql.DatabaseMetaData;
import java.sql.SQLException;
import javax.sql.DataSource;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.jpattern.orm.dialect.DBType;
import com.jpattern.orm.dialect.DetermineDBType;
/**
*
* @author Francesco Cina
*
* 21/mag/2011
*/
public abstract class SessionProvider {
private final Logger logger = LoggerFactory.getLogger(this.getClass());
private DBType dbType;
public abstract SessionStrategy getSessionStrategy();
public abstract DataSource getDataSource();
public final DBType getDBType() {
if (this.dbType==null) {
Connection connection = null;
this.dbType = DBType.UNDEFINED;
try
{
DataSource dataSource = getDataSource();
if (dataSource!=null) {
connection = dataSource.getConnection();
DatabaseMetaData metaData = connection.getMetaData();
String driverName = metaData.getDriverName();
String driverVersion = metaData.getDriverVersion();
String url = metaData.getURL();
String databaseProductName = metaData.getDatabaseProductName();
getLogger().info("DB username: " + metaData.getUserName());
getLogger().info("DB driver name: " + driverName);
getLogger().info("DB driver version: " + driverVersion);
getLogger().info("DB url: " + url);
getLogger().info("DB product name: " + databaseProductName);
getLogger().info("DB product version: " + metaData.getDatabaseProductVersion());
this.dbType = new DetermineDBType().determineDBType(driverName, url, databaseProductName);
}
}
catch (SQLException ex)
{
getLogger().warn("Error while determining the database type");
}
finally
{
if (connection != null)
{
try
{
connection.close();
}
catch (SQLException ex)
{
// we ignore this one
}
}
}
getLogger().info("DB type is " + this.dbType);
}
return this.dbType;
}
public Logger getLogger() {
return this.logger;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy