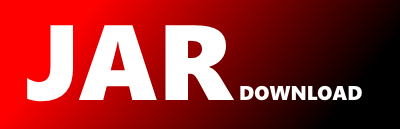
com.jporm.rx.session.Session Maven / Gradle / Ivy
The newest version!
/*******************************************************************************
* Copyright 2015 Francesco Cina'
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
******************************************************************************/
package com.jporm.rx.session;
import com.jporm.commons.core.exception.JpoException;
import com.jporm.rx.query.delete.CustomDeleteQuery;
import com.jporm.rx.query.delete.DeleteResult;
import com.jporm.rx.query.find.CustomFindQuery;
import com.jporm.rx.query.find.CustomResultFindQueryBuilder;
import com.jporm.rx.query.find.FindQuery;
import com.jporm.rx.query.save.CustomSaveQuery;
import com.jporm.rx.query.update.CustomUpdateQuery;
import io.reactivex.Single;
public interface Session {
/**
* Delete one bean from the database
*
* @param bean
* @param cascade
* @return
*/
Single delete(BEAN bean) throws JpoException;
/**
* Delete entries from a specific table
*
* @param clazz
* the TABLE related Class
* @return
*/
CustomDeleteQuery delete(Class clazz) throws JpoException;
// /**
// * Delete the beans from the database
// * @param
// * @param beans the beans to delete
// * @throws JpoException
// * @return
// */
// CompletableFuture delete(Collection beans)
// throws JpoException;
/**
* Create a new query to find bean
*
* @param
* @param clazz
* The class of the bean that will be retrieved by the query
* execution. The simple class name will be used as alias for the
* class
* @return
* @throws JpoException
*/
CustomFindQuery find(Class clazz) throws JpoException;
/**
* Create a new query to find bean
*
* @param
* @param clazz
* The class of the bean that will be retrieved by the query
* execution.
* @param alias
* The alias of the class in the query.
* @return
* @throws JpoException
*/
CustomFindQuery find(Class clazz, String alias) throws JpoException;
/**
* Create a new custom query that permits to specify a custom select clause.
*
* @param
* @param selectFields
* @return
*/
CustomResultFindQueryBuilder find(String... selectFields);
/**
* Find a bean using its ID.
*
* @param
* @param clazz
* The Class of the bean to load
* @param idValue
* the value of the identifying column of the bean
* @return
*/
FindQuery findById(Class clazz, Object idValue);
/**
* Find a bean using another bean as model. The model class and id(s) will
* be used to build the find query.
*
* @param
* @param bean
* @return
*
*/
FindQuery findByModelId(BEAN model);
/**
* Persist the new bean in the database
*
* @param
* @param bean
* @throws JpoException
* @return
*/
Single save(BEAN bean);
/**
* Permits to define a custom insert query
*
* @param clazz
* the TABLE related Class
* @throws JpoException
*/
CustomSaveQuery save(Class clazz, String... fields) throws JpoException;
// /**
// * Persist the new beans in the database
// * @param beans the beans to persist
// * @param cascade whether to persist the children recursively
// * @return
// * @throws JpoException
// */
// List save(Collection beans) throws JpoException;
/**
* Updates the bean if it exists, otherwise it saves it
*
* @param bean
* the bean to be persisted
* @return
* @throws JpoException
*/
Single saveOrUpdate(BEAN bean);
/**
* An executor to perform any kind of plain SQL statements.
*
* @return
*/
SqlSession sql();
// /**
// * Update the values of the existing beans in the database
// * @param
// * @param beans the beans to update
// * @throws JpoException
// * @return
// */
// List update(Collection beans) throws JpoException;
/**
* @param aggregatedUser
* @return
*/
Single update(BEAN bean) throws JpoException;
/**
* Update the entries of a specific TABLE
*
* @param clazz
* the TABLE related Class
* @throws JpoException
*/
CustomUpdateQuery update(Class clazz) throws JpoException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy