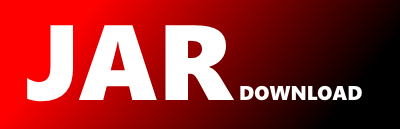
com.jporm.sql.query.select.SelectImpl Maven / Gradle / Ivy
/*******************************************************************************
* Copyright 2013 Francesco Cina'
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
******************************************************************************/
package com.jporm.sql.query.select;
import java.util.ArrayList;
import java.util.List;
import java.util.function.Supplier;
import com.jporm.sql.dialect.SqlSelectRender;
import com.jporm.sql.query.processor.PropertiesProcessor;
import com.jporm.sql.query.processor.TableName;
import com.jporm.sql.query.processor.TablePropertiesProcessor;
import com.jporm.sql.query.select.from.FromImpl;
import com.jporm.sql.query.select.groupby.SelectGroupBy;
import com.jporm.sql.query.select.groupby.SelectGroupByImpl;
import com.jporm.sql.query.select.orderby.SelectOrderByImpl;
import com.jporm.sql.query.select.pagination.SelectPaginationProvider;
import com.jporm.sql.query.select.unions.SelectUnionsProvider;
import com.jporm.sql.query.select.where.SelectWhereImpl;
/**
*
* @author Francesco Cina
*
* 07/lug/2011
*/
public class SelectImpl extends FromImpl> implements Select {
private final PropertiesProcessor propertiesProcessor;
private final SelectWhereImpl where;
private final SelectOrderByImpl orderBy;
private final SelectGroupByImpl groupBy;
private final List unions = new ArrayList<>();
private final List unionAlls = new ArrayList<>();
private final List intersects = new ArrayList<>();
private final List excepts = new ArrayList<>();
private boolean distinct = false;
private LockMode lockMode = LockMode.NO_LOCK;
private int maxRows = 0;
private int firstRow = -1;
private final Supplier selectFields;
private final SqlSelectRender selectRender;
public SelectImpl(SqlSelectRender selectRender, Supplier selectFields, final TYPE tableNameSource, final TablePropertiesProcessor propertiesProcessor) {
this(selectRender, selectFields, propertiesProcessor.getTableName(tableNameSource), propertiesProcessor);
}
public SelectImpl(SqlSelectRender selectRender, Supplier selectFields, final TYPE tableNameSource, final TablePropertiesProcessor propertiesProcessor, final String alias) {
this(selectRender, selectFields, propertiesProcessor.getTableName(tableNameSource, alias), propertiesProcessor);
}
private SelectImpl(SqlSelectRender selectRender, Supplier selectFields, final TableName tableName, final TablePropertiesProcessor propertiesProcessor) {
super(tableName, propertiesProcessor);
this.selectFields = selectFields;
this.propertiesProcessor = propertiesProcessor;
this.selectRender = selectRender;
where = new SelectWhereImpl(this);
orderBy = new SelectOrderByImpl(this);
groupBy = new SelectGroupByImpl(this);
}
@Override
public final void sqlValues(final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy