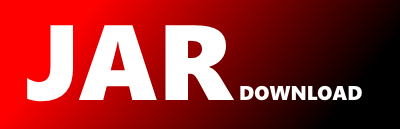
com.jporm.test.BaseTestAllDB Maven / Gradle / Ivy
/*******************************************************************************
* Copyright 2013 Francesco Cina'
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
******************************************************************************/
package com.jporm.test;
import java.math.BigDecimal;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Date;
import java.util.List;
import java.util.Map.Entry;
import org.junit.After;
import org.junit.Before;
import org.junit.Rule;
import org.junit.rules.TestName;
import org.junit.runner.RunWith;
import org.junit.runners.Parameterized;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.context.ApplicationContext;
import org.springframework.context.annotation.AnnotationConfigApplicationContext;
import com.jporm.core.JPOrm;
import com.jporm.test.config.DBData;
/**
*
* @author Francesco Cina
*
* 20/mag/2011
*/
@RunWith(Parameterized.class)
//BaseTestAllDB
public abstract class BaseTestAllDB {
public static ApplicationContext CONTEXT = null;
private final TestData testData;
public BaseTestAllDB(final String testName, final TestData testData) {
this.testData = testData;
}
@Parameterized.Parameters(name="{0}")
public static Collection
© 2015 - 2025 Weber Informatics LLC | Privacy Policy