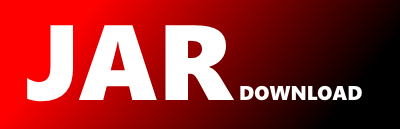
com.jquicker.model.BaseEntity Maven / Gradle / Ivy
package com.jquicker.model;
import java.io.Serializable;
import java.lang.reflect.Field;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.lang.reflect.Modifier;
import java.lang.reflect.ParameterizedType;
import java.lang.reflect.Type;
import java.util.HashMap;
import java.util.Map;
import com.alibaba.fastjson.JSONObject;
import com.jquicker.annotation.beans.Entity;
import com.jquicker.annotation.beans.Transient;
import com.jquicker.commons.util.JsonUtils;
import com.jquicker.commons.util.StringUtils;
@Entity
public class BaseEntity extends JQuickerMap implements Serializable {
private static final long serialVersionUID = 1L;
/**
* 设实体对象置属性值
* @param key
* @param value
* @author OL
*/
public void set(String key, Object value) {
try {
Class extends BaseEntity> clazz = this.getClass();
Field field = clazz.getDeclaredField(key);
field.setAccessible(true);
field.set(this, value);
} catch (NoSuchFieldException | IllegalArgumentException | IllegalAccessException e) {
super.put(key, value);
}
}
/**
* 从实体对象中取属性值
* 注:此方法优先取出存储在Map对象中的值
*/
@SuppressWarnings("unchecked")
public T get(String key) {
if(super.containsKey(key)) {
return (T) super.get(key);
}
try {
Class extends BaseEntity> clazz = this.getClass();
Field field = clazz.getDeclaredField(key);
field.setAccessible(true);
return (T) field.get(this);
} catch (Exception e) {
e.printStackTrace();
}
return null;
}
public JSONObject toJSON() {
JSONObject object = new JSONObject(this);
Class extends BaseEntity> clazz = this.getClass();
Field[] fields = clazz.getDeclaredFields();
try {
for (Field field : fields) {
if(field.isAnnotationPresent(Transient.class)) {
continue;
}
String name = field.getName();
if(name.equals("serialVersionUID")) {
continue;
}
field.setAccessible(true);
Object value = field.get(this);
object.put(name, value);
}
} catch (IllegalArgumentException | IllegalAccessException e) {
e.printStackTrace();
}
return object;
}
/**
* 转换成BaseEntity
* @param map
* @param clazz
* @return
* @author OL
*/
public T toEntity(Class clazz) {
try {
T entity = clazz.newInstance();
Method[] methods = clazz.getDeclaredMethods();
for (Method method : methods) {
String methodName = method.getName();
if(method.getModifiers() == Modifier.PUBLIC && methodName.startsWith("set")){
String fieldName = StringUtils.toLowerCaseFirstLetter(methodName.substring(3));
try {
method.invoke(entity, this.remove(fieldName));
} catch (IllegalAccessException | IllegalArgumentException | InvocationTargetException e) {
e.printStackTrace();
}
}
}
entity.putAll(this);
entity.toString(); // 不toString一下,前端无法取到值 ??? TODO
return entity;
} catch (Exception e) {
e.printStackTrace();
}
return null;
}
/**
* 获取自定义的属性
* 注:BaseEntity继承自HashMap,因此除了其自身定义的“原始属性”外还可以存储额外的属性
* @return
* @author OL
*/
public Map getDefinedFields(){
Map map = new HashMap();
Class extends BaseEntity> clazz = this.getClass();
Field[] fields = clazz.getDeclaredFields();
try {
for (Field field : fields) {
if(field.isAnnotationPresent(Transient.class)) {
continue;
}
String name = field.getName();
if(name.equals("serialVersionUID")) {
continue;
}
field.setAccessible(true);
Object value = field.get(this);
map.put(name, value);
}
} catch (IllegalArgumentException | IllegalAccessException e) {
e.printStackTrace();
}
return map;
}
/**
* 获取所有的属性
* 包括自定义属性和存储在Map中的属性
* @return
* @author OL
*/
public Map getFields(){
Map map = new HashMap(this);
Class extends BaseEntity> clazz = this.getClass();
Field[] fields = clazz.getDeclaredFields();
try {
for (Field field : fields) {
/*if(field.isAnnotationPresent(Transient.class)) {
continue;
}*/
String name = field.getName();
if(name.equals("serialVersionUID")) {
continue;
}
field.setAccessible(true);
Object value = field.get(this);
map.put(name, value);
}
} catch (IllegalArgumentException | IllegalAccessException e) {
e.printStackTrace();
}
return map;
}
public boolean isField(String name) {
Class extends BaseEntity> clazz = this.getClass();
try {
clazz.getDeclaredField(name);
return true;
} catch (NoSuchFieldException e) {
return false;
} catch (SecurityException e) {
return false;
}
}
/**
* 将Map转换成Entity
* @param map
* @param clazz
* @return
* @author OL
*/
public static T toEntity(Map map, Class clazz) {
if(map == null) {
return null;
}
try {
T entity = clazz.newInstance();
Method[] methods = clazz.getDeclaredMethods();
for (Method method : methods) {
String methodName = method.getName();
if(method.getModifiers() == Modifier.PUBLIC && methodName.startsWith("set")){
String fieldName = StringUtils.toLowerCaseFirstLetter(methodName.substring(3));
try {
method.invoke(entity, map.remove(fieldName));
} catch (IllegalAccessException | IllegalArgumentException | InvocationTargetException e) {
e.printStackTrace();
}
}
}
/*Field[] fields = clazz.getDeclaredFields();
for (Field field : fields) {
String fieldName = field.getName();
if(fieldName.equals("serialVersionUID")) {
continue;
}
boolean accessible = field.isAccessible();
field.setAccessible(true);
try {
field.set(entity, map.remove(fieldName));
field.setAccessible(accessible);
} catch (Exception e) {
e.printStackTrace();
}
}*/
entity.toString(); // 不toString一下,前端无法取到值 ??? TODO
if(BaseEntity.class.isAssignableFrom(clazz)){
((BaseEntity) entity).putAll(map);
}
return entity;
} catch (Exception e) {
e.printStackTrace();
}
return null;
}
/**
* 取得实体对象的主键以及值 TODO
* @return
* @author OL
*/
public String[] getPrimaryKey() {
return null;
}
/**
* 获取某个属性的类型
* @return
* @author OL
*/
public Class> getFieldType(String fieldName) {
Class extends BaseEntity> clazz = this.getClass();
try {
Field field = clazz.getDeclaredField(fieldName);
return field.getType();
} catch (NoSuchFieldException | SecurityException e) {
}
return null;
}
/**
* 获取某个属性的类型
*
* @return
* @author OL
*/
public Class> getGenericType(String fieldName) {
Class extends BaseEntity> clazz = this.getClass();
try {
Field field = clazz.getDeclaredField(fieldName);
Type genericType = field.getGenericType();
// 如果是泛型参数的类型
if(genericType instanceof ParameterizedType){
ParameterizedType pt = (ParameterizedType) genericType;
//得到泛型里的class类型对象
Class> accountPrincipalApproveClazz = (Class>)pt.getActualTypeArguments()[0];
return accountPrincipalApproveClazz;
}
} catch (NoSuchFieldException | SecurityException e) {
}
return null;
}
@Override
public boolean isEmpty() {
Map map = new HashMap(this);
Class extends BaseEntity> clazz = this.getClass();
Field[] fields = clazz.getDeclaredFields();
try {
for (Field field : fields) {
String name = field.getName();
if(name.equals("serialVersionUID")) {
continue;
}
field.setAccessible(true);
Object value = field.get(this);
if(value == null) {
continue;
}
map.put(name, value);
}
} catch (IllegalArgumentException | IllegalAccessException e) {
e.printStackTrace();
}
return map.isEmpty();
}
@Override
public String toString() {
// return JsonUtils.toJsonString(this);
return JsonUtils.toJsonString(toJSON());
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy