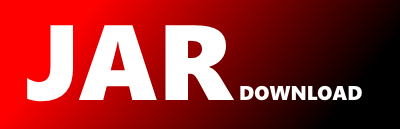
com.jquicker.model.JQuickerMap Maven / Gradle / Ivy
package com.jquicker.model;
import java.math.BigDecimal;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import com.jquicker.commons.util.JsonUtils;
import com.jquicker.commons.util.NumberUtils;
public class JQuickerMap extends HashMap {
private static final long serialVersionUID = 5584732871601633358L;
@SuppressWarnings("unchecked")
public T get(String key) {
return (T) super.get(key);
}
public Short getShort(String key) {
return NumberUtils.parseToShort(super.get(key));
}
public short getShortValue(String key) {
return this.getShortValue(key, (short) 0);
}
public short getShortValue(String key, short defaultValue) {
return NumberUtils.parseToShort(super.get(key), defaultValue);
}
public Integer getInteger(String key) {
return NumberUtils.parseToInteger(super.get(key));
}
public int getIntValue(String key) {
return this.getIntValue(key, 0);
}
public int getIntValue(String key, int defaultValue) {
return NumberUtils.parseToInteger(super.get(key), defaultValue);
}
public Long getLong(String key) {
return NumberUtils.parseToLong(super.get(key));
}
public long getLongValue(String key) {
return this.getLongValue(key, 0);
}
public long getLongValue(String key, long defaultValue) {
return NumberUtils.parseToLong(super.get(key), defaultValue);
}
public Double getDouble(String key) {
return NumberUtils.parseToDouble(super.get(key));
}
public double getDoubleValue(String key) {
return NumberUtils.parseToDouble(super.get(key), 0D);
}
public double getDoubleValue(String key, double defaultValue) {
return NumberUtils.parseToDouble(super.get(key), defaultValue);
}
public Float getFloat(String key) {
return NumberUtils.parseToFloat(super.get(key));
}
public float getFloatValue(String key) {
return NumberUtils.parseToFloat(super.get(key), 0F);
}
public float getFloatValue(String key, float defaultValue) {
return NumberUtils.parseToFloat(super.get(key), defaultValue);
}
public BigDecimal getBigDecimal(String key) {
if (super.containsKey(key)) {
Object obj = super.get(key);
if (NumberUtils.isNumerical(obj)) {
return new BigDecimal(obj.toString());
}
}
return null;
}
public BigDecimal getBigDecimal(String key, int scale) {
return this.getBigDecimal(key, scale, BigDecimal.ROUND_HALF_UP); // 四舍五入
}
public BigDecimal getBigDecimal(String key, int scale, int roundingMode) {
BigDecimal bigDecimal = this.getBigDecimal(key);
if (bigDecimal != null) {
return bigDecimal.setScale(scale, roundingMode);
}
return bigDecimal;
}
public boolean getBooleanValue(String key, boolean defaultValue) {
if (super.containsKey(key)) {
Object obj = super.get(key);
if (obj != null) {
return Boolean.valueOf(obj.toString());
}
}
return defaultValue;
}
public boolean getBooleanValue(String key) {
return this.getBooleanValue(key, false);
}
public Boolean getBoolean(String key) {
if (super.containsKey(key)) {
Object obj = super.get(key);
if (obj != null) {
return Boolean.valueOf(obj.toString());
}
}
return null;
}
public String getString(String key, String defaultValue) {
if (super.containsKey(key)) {
Object obj = super.get(key);
if (obj != null) {
return obj.toString();
}
}
return defaultValue;
}
public String getString(String key) {
return this.getString(key, null);
}
@SuppressWarnings("unchecked")
public List getList(String key) {
return (List) super.get(key);
}
@Override
public String toString() {
return JsonUtils.toJsonString(this);
}
public static void main(String[] args) {
JQuickerMap map = new JQuickerMap();
map.put("obj1", new String("sss"));
String s = map.get("obj1");
System.out.println(s);
BigDecimal bd = new BigDecimal("11112314545645446");
System.out.println(bd.intValue());
Map map1 = new HashMap();
map1.put("a", "1");
map.put("map1", map1);
Map obj = map.get("map1");
System.out.println(obj);
Integer i = obj.get("a");
System.out.println(i);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy