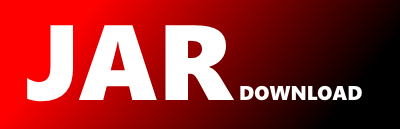
com.jquicker.persistent.rdb.sqlfile.SQLManager Maven / Gradle / Ivy
package com.jquicker.persistent.rdb.sqlfile;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileReader;
import java.io.IOException;
import java.io.InputStream;
import java.util.Enumeration;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.jar.JarEntry;
import java.util.jar.JarFile;
import com.jquicker.commons.util.IOUtils;
import com.jquicker.commons.util.PathUtils;
import com.jquicker.commons.util.StringUtils;
import com.jquicker.model.JQuickerMap;
import com.jquicker.persistent.rdb.model.DynamicSQL;
import com.jquicker.persistent.rdb.model.Relation;
import com.jquicker.persistent.rdb.model.SQLFile;
/**
* SQL文件管理类
* 默认在项目classpath目录下查找所有.sql文件
* @author OL
*/
public class SQLManager {
private static Map sqlFileMap = new JQuickerMap();
private static String sqlPath = PathUtils.getClasspath();
private SQLManager() {
}
public static void setSQLPath(String sqlPath) {
SQLManager.sqlPath = sqlPath;
init();
}
public static SQLFile getSQLFile(String namespace) {
if(sqlFileMap.isEmpty()) {
init();
}
return sqlFileMap.get(namespace);
}
public static DynamicSQL getSQL(String namespace, String sqlId) {
if(sqlFileMap.isEmpty()) {
init();
}
SQLFile sqlFile = sqlFileMap.get(namespace);
if(sqlFile == null) {
return null;
}
return sqlFile.getSql(sqlId);
}
public static void init() {
File rootFile = new File(sqlPath);
if (rootFile.isFile()) {
String fileName = rootFile.getName();
if(fileName.endsWith(".sql")) {
processSqlFile(rootFile);
} else if(fileName.endsWith(".jar")){
processJar(rootFile);
}
} else {
processDirectory(rootFile);
}
}
private static void processSqlFile(File sqlFile) {
readFileByLines(sqlFile);
}
private static void processDirectory(File directory) {
File[] files = directory.listFiles();
if(files == null) {
return;
}
for (File file : files) {
if (file.isFile()) {
String fileName = file.getName();
if(fileName.endsWith(".sql")) {
processSqlFile(file);
} else if(fileName.endsWith(".jar")){
processJar(file);
}
} else {
processDirectory(file);
}
}
}
/**
* 读取Jar包中的sql文件 TODO
* @param file
* @author OL
*/
private static void processJar(File file) {
try {
JarFile jarFile = new JarFile(file);
Enumeration jarEntrys = jarFile.entries();
while (jarEntrys.hasMoreElements()) {
JarEntry jarEntry = jarEntrys.nextElement();
String name = jarEntry.getName();
if (name.endsWith(".sql")) {
InputStream is = jarFile.getInputStream(jarEntry);
String result = IOUtils.read(is); // TODO
System.out.println(result); // TODO
}
}
jarFile.close();
} catch (IOException e) {
e.printStackTrace();
}
}
/**
* 以行为单位读取文件
*/
private static void readFileByLines(File file) {
BufferedReader reader = null;
try {
reader = new BufferedReader(new FileReader(file));
int line = 1;
String tmp = null;
SQLFile sqlFile = null;
StringBuilder sqlBuilder = null;
String sqlId = null;
while ((tmp = reader.readLine()) != null) {
if(StringUtils.isEmpty(tmp.trim())) {
continue;
}
if(line == 1) {
String namespace = SQLFile.isSqlFile(tmp);
if(namespace == null) {
continue;
}
sqlFile = new SQLFile(namespace);
sqlFileMap.put(namespace, sqlFile);
} else {
if(StringUtils.isEmpty(tmp)) {
continue;
}
if(tmp.startsWith("--")) {
continue;
}
// 处理${variable}参数引用
tmp = replaceVariables(tmp, sqlFile.getVariables());
String sqlInfo = SQLFile.isSql(tmp);
if(sqlInfo != null) {
if(sqlBuilder != null && sqlBuilder.length() > 0) { // sqlBuilder不为空表示上一条Sql到本行之前已经结束了
DynamicSQL sql = sqlFile.getSql(sqlId);
sql.setSqlStatement(sqlBuilder.toString().trim());
}
Map map = new HashMap();
if(sqlInfo.indexOf("=") > 0) {
String[] infos = sqlInfo.split(",");
for (String info : infos) {
String[] array = info.trim().split("=");
map.put(array[0], array[1].replace("\"", "")); // 去掉双引号
}
} else {
sqlId = sqlInfo;
map.put("id", sqlInfo.replace("\"", ""));
}
sqlId = map.get("id");
DynamicSQL sql = new DynamicSQL(sqlId);
sql.setParamType(map.get("paramType"));
Class> clazz = Object.class;
String returnType = map.get("returnType");
if(StringUtils.isNotEmpty(returnType)){
try {
clazz = Class.forName(returnType);
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
}
sql.setReturnType(clazz);
sqlFile.addSql(sqlId, sql);
sqlBuilder = new StringBuilder(); // 将sqlBuilder清空,以便拼装接下来的sql语句内容
} else {
// 判断是否relation
String relationInfo = SQLFile.isRelation(tmp);
if(relationInfo != null) {
String[] infos = relationInfo.split(",");
Map map = new HashMap();
for (String info : infos) {
String[] array = info.trim().split("=");
map.put(array[0], array[1].replace("\"", "")); // 去掉双引号
}
Relation relation = new Relation();
relation.setColumns(map.get("columns"));
relation.setProperty(map.get("property"));
relation.setQuery(map.get("query"));
String namespace = map.get("namespace");
relation.setNamespace(StringUtils.isEmpty(namespace) ? sqlFile.getNamespace() : namespace); // 缺省为当前namespace
relation.setType(map.get("type"));
DynamicSQL sql = sqlFile.getSql(sqlId);
sql.addRelation(relation);
continue;
}
String mapping = SQLFile.isMapping(tmp);
if(mapping != null) {
sqlFile.setMapping(mapping);
continue;
}
Map variable = SQLFile.isVariable(tmp);
if(variable != null){
sqlFile.addVariable(variable);
continue;
}
if(sqlBuilder != null) {
sqlBuilder.append(tmp);
}
}
}
// System.out.println(tmp);
if(sqlBuilder != null && sqlBuilder.length() > 0 && !sqlBuilder.toString().endsWith(" ")) {
sqlBuilder.append(" ");
}
line++;
}
if(sqlBuilder != null && sqlBuilder.length() > 0) {
DynamicSQL sql = sqlFile.getSql(sqlId);
sql.setSqlStatement(sqlBuilder.toString().trim());
}
reader.close();
} catch (IOException e) {
e.printStackTrace();
} finally {
if (reader != null) {
try {
reader.close();
} catch (IOException e1) {
}
}
}
}
/**
* 处理${variable}
* @param content
* @return
*/
private static String replaceVariables(String content, Map variables){
List list = SQLFile.getVariable(content);
for (String variable : list) {
content = content.replace("${" + variable + "}", variables.get(variable));
}
return content;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy