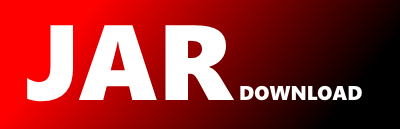
com.jquicker.persistent.rdb.util.ResultSetUtils Maven / Gradle / Ivy
package com.jquicker.persistent.rdb.util;
import java.math.BigDecimal;
import java.sql.ResultSet;
import java.sql.ResultSetMetaData;
import java.sql.SQLException;
import java.sql.Timestamp;
import java.sql.Types;
import java.util.ArrayList;
import java.util.Date;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
import com.jquicker.commons.util.ObjectUtils;
import com.jquicker.model.BaseEntity;
import com.jquicker.model.ResultMap;
/**
* java.sql.ResultSet工具类
*
* @author OL
*/
public class ResultSetUtils {
/**
* 将ResultSet结果集转换成Map
* 列名与结果集数据分开存储,适用于处理大量数据的结果集
*
* @param resultSet
* @param moreMeta
* @return
* @throws SQLException
* @author OL
*/
public static ResultMap convert(ResultSet resultSet, boolean moreMeta) {
ResultMap map = new ResultMap();
List fields = new ArrayList();
List> fieldList = moreMeta ? new ArrayList>() : null;
List> records = new ArrayList>();
try {
ResultSetMetaData metaData = resultSet.getMetaData();
int colCount = metaData.getColumnCount();
for (int i = 1; i <= colCount; i++) {
String name = metaData.getColumnLabel(i);
fields.add(name);
if (moreMeta) {
ResultMap field = new ResultMap();
field.put("name", name);
field.put("size", metaData.getColumnDisplaySize(i));
field.put("precision", metaData.getPrecision(i));
field.put("scale", metaData.getScale(i));
field.put("type", metaData.getColumnType(i));
String typeName = metaData.getColumnTypeName(i);
if (name.startsWith("lob_") && typeName.equalsIgnoreCase("varchar2"))
field.put("typeName", "blob");
else
field.put("typeName", typeName);
fieldList.add(field);
}
}
while (resultSet.next()) {
List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy