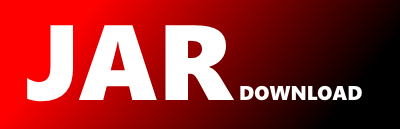
com.jquicker.timer.TimerConfig Maven / Gradle / Ivy
package com.jquicker.timer;
import java.io.IOException;
import java.io.InputStream;
import java.lang.reflect.Method;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Map;
import org.dom4j.Document;
import org.dom4j.DocumentException;
import org.dom4j.Element;
import org.dom4j.io.SAXReader;
import com.jquicker.commons.util.NumberUtils;
public class TimerConfig {
private static String CONFIG_TASK_ID = "configerTask"; // 定时加载配置文件的任务
private static String CONFIG_TASK_CLASS = "com.jquicker.timer.ConfigTask";
private static String CONFIG_TASK_METHOD = "reload";
private static boolean AUTO_RELOAD = false;
private static long MONITOR_INTERVAL = 1800; // 默认30min重新加载
public static synchronized void init(String configPath) {
InputStream inputStream = TimerConfig.class.getResourceAsStream(configPath);
SAXReader reader = new SAXReader();
Document document = null;
try {
document = reader.read(inputStream);
Element root = document.getRootElement();
AUTO_RELOAD = Boolean.valueOf(root.attributeValue("autoReload"));
TimerTask sysTask = new TimerTask();
sysTask.setId(CONFIG_TASK_ID);
Class> clazz = Class.forName(CONFIG_TASK_CLASS);
Method method = clazz.getDeclaredMethod(CONFIG_TASK_METHOD);
sysTask.setClazz(clazz);
sysTask.setMethod(method);
sysTask.setTask(new ConfigTask(configPath));
if(AUTO_RELOAD){
MONITOR_INTERVAL = NumberUtils.parseToLong(root.attributeValue("monitorInterval"), MONITOR_INTERVAL);
sysTask.setPeriod(MONITOR_INTERVAL);
}
TimerManager.add(sysTask);
Iterator> iterator = root.elementIterator();
while (iterator.hasNext()) {
Element ele = (Element) iterator.next();
TimerTask timerInfo = new TimerTask();
timerInfo.setId(ele.attributeValue("id"));
Iterator> it = ele.elementIterator();
Map tmp = new HashMap();
while (it.hasNext()) {
Element element = (Element) it.next();
String key = element.attributeValue("name");
String value = element.attributeValue("value");
if("cronExpression".equals(key)){
CronUtils.check(value);
}
tmp.put(key, value);
}
if(!tmp.containsKey("class")){
throw new RuntimeException("Config Error: Class not configured");
}
if(!tmp.containsKey("method")){
throw new RuntimeException("Config Error: Method not configured");
}
Class> c = Class.forName(tmp.get("class"));
Method m = clazz.getDeclaredMethod(tmp.get("mathod"));
timerInfo.setName(tmp.get("name"));
timerInfo.setClazz(c);
timerInfo.setMethod(m);
timerInfo.setCron(tmp.get("cron"));
timerInfo.setFirst(tmp.get("first"));
timerInfo.setConcurrent(Boolean.valueOf(tmp.get("concurrent")));
// timerInfo.setPolicy(tmp.get("policy"));
timerInfo.setDescription(tmp.get("description"));
TimerManager.add(timerInfo);
}
} catch (DocumentException | ClassNotFoundException | NoSuchMethodException e) {
throw new RuntimeException(e);
} finally {
try {
if(inputStream != null){
inputStream.close();
}
} catch (IOException e) {
}
}
}
public static void main(String[] args) {
TimerConfig.init("/timer.xml");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy