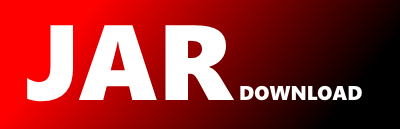
com.jquicker.timer.TimerManager Maven / Gradle / Ivy
package com.jquicker.timer;
import java.util.ArrayList;
import java.util.Date;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.concurrent.Executors;
import java.util.concurrent.ScheduledExecutorService;
import java.util.concurrent.ScheduledFuture;
import java.util.concurrent.TimeUnit;
import com.jquicker.annotation.timer.Scheduled.Policy;
import com.jquicker.commons.log.JLogger;
import com.jquicker.commons.util.DateUtils;
import com.jquicker.commons.util.StringUtils;
import com.jquicker.configure.Config;
/**
* 定时任务管理类
*
* @author OL
*
*/
public class TimerManager {
private static final TimeUnit unit = TimeUnit.SECONDS;
private static final int corePoolSize = Config.getInt("timer.corePoolSize", 2);
private static ScheduledExecutorService exec = Executors.newScheduledThreadPool(corePoolSize);
private static Map tasks = new HashMap();
private static Map> futures = new HashMap>();
public static void add(TimerTask timerTask) {
String id = timerTask.getId();
if(tasks.containsKey(id)){
throw new RuntimeException("任务ID重复:" + id);
}
tasks.put(id, timerTask);
}
public static void start() {
JLogger.debug("Timer initialize start.");
Iterator it = tasks.keySet().iterator();
ScheduledFuture> future = null;
while (it.hasNext()) {
String id = (String) it.next();
TimerTask timer = tasks.get(id);
if(!timer.isEnable()){
continue;
}
long period = timer.getPeriod();
long initialDelay = 0;
String first = timer.getFirst();
if(StringUtils.isNotEmpty(first)){
Date firstDate = null;
if(first.length() > 8){
firstDate = DateUtils.parse(first, DateUtils.TO_SECOND_LINE);
} else if(first.length() == 8){
firstDate = DateUtils.parse(first, DateUtils.ONLY_TIME_COLON);
}
firstDate = firstDate == null ? new Date() : firstDate;
long firstTime = firstDate.getTime();
long nowTime = DateUtils.nowTime();
// System.out.println(DateUtils.now(DateUtils.ONLY_TIME_COLON));
if(nowTime <= firstTime){
initialDelay = (firstTime - nowTime) / 1000;
} else {
// long n = (nowTime - firstTime) / (period * 1000); // 已过去的周期数
// initialDelay = (firstTime + (n + 1) * period * 1000 - nowTime) / 1000;
// System.out.println(initialDelay);
initialDelay = period - (nowTime - firstTime) / 1000 % period;
// System.out.println(initialDelay);
}
}
TimerCommand command = null;
if(timer.getTask() == null){
command = new TimerCommand(id, timer.getMethod(), timer.getClazz());
} else {
command = new TimerCommand(id, timer.getMethod(), timer.getTask());
}
if(timer.isCyclical()){
Policy policy = timer.getPolicy();
if(policy == Policy.atFixedRate){
// 以上一个任务开始的时间计时,period时间过去后,检测上一个任务是否执行完毕
future = exec.scheduleAtFixedRate(command, initialDelay, period, unit);
} else {
// 以上一个任务结束时开始计时,period时间过去后,立即执行
future = exec.scheduleWithFixedDelay(command, initialDelay, period, unit);
}
} else {
future = exec.schedule(command, timer.getDelay(), unit); // 非周期的任务
}
futures.put(id, future);
JLogger.debug("Timer [{}] initialized.", id);
}
JLogger.debug("Timer initialize completed.");
}
/**
* 暂停
* @param key
* @return
* @author OL
*/
public static boolean pause(String key) {
ScheduledFuture> future = futures.get(key);
if (future != null) {
synchronized(future){
// 如果参数为true并且任务正在运行,那么这个任务将被取消
// 如果参数为false并且任务正在运行,那么这个任务将不会被取消
return future.cancel(false);
}
}
return false;
}
/**
* 恢复
* @param key
* @return
* @author OL
*/
public static boolean resume(String key) {
ScheduledFuture> future = futures.get(key);
if (future != null) {
synchronized(future){
future.notify();
return true;
}
}
return false;
}
/**
* 删除
* @param key
* @return
* @author OL
*/
public static boolean remove(String key) {
ScheduledFuture> future = futures.get(key);
if (future != null) {
synchronized(key){
if (future.cancel(true)) {
futures.remove(key);
return true;
}
}
}
return false;
}
public static Map> getFutures(){
return futures;
}
public static List getTimerTasks(){
List list = new ArrayList(tasks.values());
return list;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy