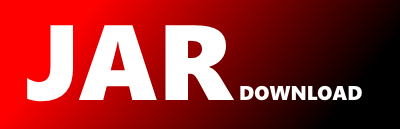
com.jrodeo.bson.marshallers.MarshallerRegistry Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of bson-marshaller Show documentation
Show all versions of bson-marshaller Show documentation
A tool to create Java marshallers to and from bson. Currently used in a data access
layer serialize Json to Bson for MongoDB storage.
package com.jrodeo.bson.marshallers;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* This class is responsbile for runtime management of the factories and marshallers. It is used
* during marshalling to lookup code to perform the tasks of serializing.
*/
public class MarshallerRegistry {
private static final MarshallerRegistry instance = new MarshallerRegistry();
static {
instance.registerFactory(new DefaultMarshallerFactory());
}
public static MarshallerRegistry getInstance() {
return instance;
}
MarshallerRegistry() {
}
Map classToMarshaller = new HashMap<>();
Map> superToSubTypeMarshaller = new HashMap<>();
public void registerFactory(MarshallerFactory factory) {
for (Marshaller marshaller : factory.getMarshallers()) {
marshaller.setRegistry(this);
Class clazz = marshaller.getForClass();
classToMarshaller.put(clazz, marshaller);
String subTypeName = marshaller.subTypeName();
if (subTypeName != null) {
Map nameToMarshaller = superToSubTypeMarshaller.get(marshaller.getSuperType());
if (nameToMarshaller == null) {
nameToMarshaller = new HashMap<>();
superToSubTypeMarshaller.put(marshaller.getSuperType(), nameToMarshaller);
}
nameToMarshaller.put(subTypeName, marshaller);
}
}
}
public void registerFactories(List factoryList) {
for (MarshallerFactory factory : factoryList) {
registerFactory(factory);
}
}
public Marshaller lookupMarshaller(Class clazz)
throws RuntimeException {
Marshaller m = classToMarshaller.get(clazz);
return m;
}
public Marshaller lookupMarshaller(T obj)
throws RuntimeException {
return lookupMarshaller(obj.getClass());
}
public Marshaller lookupMarshaller(Class parent, String subTypeName) {
Map nameToMarshaller = superToSubTypeMarshaller.get(parent);
if (nameToMarshaller != null) {
return nameToMarshaller.get(subTypeName);
}
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy