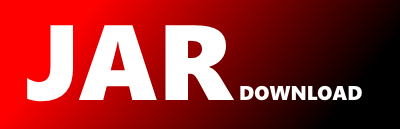
com.jrodeo.bson.marshallers.UUIDMarshaller Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of bson-marshaller Show documentation
Show all versions of bson-marshaller Show documentation
A tool to create Java marshallers to and from bson. Currently used in a data access
layer serialize Json to Bson for MongoDB storage.
package com.jrodeo.bson.marshallers;
import org.bson.BsonBinary;
import org.bson.BsonBinarySubType;
import org.bson.BsonReader;
import org.bson.BsonWriter;
import java.nio.ByteBuffer;
import java.util.UUID;
/**
* A hand coded Marshaller
to serialize java.util.UUID
.
* Note: the bson is setup to work with BsonBinarySubType.UUID_STANDARD
* for MongoDB.
*/
public class UUIDMarshaller extends AbstractSimpleMarshaller {
@Override
public Class getForClass() {
return UUID.class;
}
public void write(BsonWriter writer, UUID value) {
byte[] bytes = new byte[16];
ByteBuffer byteBuffer = ByteBuffer.wrap(bytes);
byteBuffer.putLong(value.getMostSignificantBits());
byteBuffer.putLong(value.getLeastSignificantBits());
BsonBinary binary = new BsonBinary(BsonBinarySubType.UUID_STANDARD,
bytes);
writer.writeBinaryData(binary);
}
public UUID read(BsonReader reader) {
BsonBinary binary = reader.readBinaryData();
byte[] bytes = binary.getData();
ByteBuffer byteBuffer = ByteBuffer.wrap(bytes);
UUID uuid = new UUID(byteBuffer.getLong(), byteBuffer.getLong());
return uuid;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy