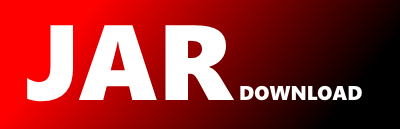
js.dom.NamespaceContext Maven / Gradle / Ivy
The newest version!
package js.dom;
import java.util.Iterator;
/**
* Abstract implementation for Java XML name space context. This class implements null methods and allows to override only the
* actual needed ones.
*
* XML name space context is used in conjunction with XPath evaluation when expression contains name space prefixes. A XML
* document may contain multiple name spaces and is possible to have XPath expression with multiple prefixes, for example
* books:booklist/science:book
. On the other hand evaluation process needs the name space URI that is the only way
* to identify the name space since the prefix is document / user specific. This class is uses to resolve name space prefixes to
* its mapped URI.
*
* In sample code books
prefix is mapped to BOOKS_URI
and science
prefix to
* SCIENCE_URI
name space URI.
*
*
* Element el = doc.getByXPathNS(new NamespaceContext() {
* @Override
* public String getNamespaceURI(String prefix) {
* if (prefix.equals("books")) {
* return BOOKS_URI;
* }
* if (prefix.equals("science")) {
* return SCIENCE_URI;
* }
* return null;
* }
* }, "books:booklist/science:book");
*
*
* @author Iulian Rotaru
* @version final
*/
public abstract class NamespaceContext implements javax.xml.namespace.NamespaceContext {
/**
* Get namespace URI bound to a prefix in the current scope.
*
* @param prefix prefix to look up.
* @return namespace URI bound to prefix in the current scope.
* @throws IllegalArgumentException if prefix
is null.
*/
@Override
public String getNamespaceURI(String prefix) {
return null;
}
/**
* Get prefix bound to namespace URI in the current scope.
*
* @param namespaceURI URI of namespace to lookup.
* @return prefix bound to namespace URI in current context.
* @throws IllegalArgumentException if namespaceURI
is null.
*/
@Override
public String getPrefix(String namespaceURI) {
return null;
}
/**
* Get all prefixes bound to a namespace URI in the current scope.
*
* @param namespaceURI URI of namespace to lookup.
* @return iterator for all prefixes bound to the namespace URI in the current scope.
* @throws IllegalArgumentException if namespaceURI
is null.
*/
@Override
public Iterator> getPrefixes(String namespaceURI) {
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy