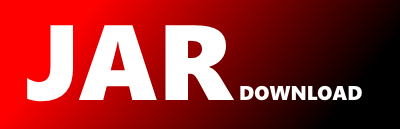
com.jsftoolkit.base.ComponentHelp Maven / Gradle / Ivy
package com.jsftoolkit.base;
import java.util.Iterator;
import javax.el.ValueExpression;
import javax.faces.component.UIComponent;
import javax.faces.component.UIComponentBase;
import javax.faces.context.FacesContext;
import com.jsftoolkit.utils.Utils;
/**
* This class may be used as a base class for {@link UIComponent}s, but will
* typically be used statically, so that components can inherit from other
* components.
*
* @author noah
*
*/
public abstract class ComponentHelp extends UIComponentBase {
/**
* Member version.
*
* @see {@link #getAttribute(Object, Object, String, UIComponent, FacesContext)
*
* @param
* @param value
* @param attribute
* @return
*/
protected T getAttribute(T value, String attribute) {
return getAttribute(value, attribute, this, getFacesContext());
}
/**
* Member version.
*
* @see {@link #getAttribute(Object, Object, String, UIComponent, FacesContext)
*
* @param
* @param value
* @param defaultValue
* @param attribute
* @return
*/
protected T getAttribute(T value, T defaultValue, String attribute) {
return getAttribute(value, defaultValue, attribute, this,
getFacesContext());
}
/**
* Get the named attribute, defaulting to null.
*
* @see {@link #getAttribute(Object, Object, String, UIComponent, FacesContext)
*
* @param
* @param value
* @param attribute
* @param component
* @param context
* @return
*/
public static T getAttribute(T value, String attribute,
UIComponent component, FacesContext context) {
return getAttribute(value, null, attribute, component, context);
}
/**
* Helper for component getters. The passed in value should be a component
* field that is set by the setter for the property. If value is non-null,
* it will always be returned. If it is null, a {@link ValueExpression} for
* the attribute will be evaluated. If that value is null, defaultValue is
* returned, otherwise the value obtained from the value expression will be
* returned.
*
* @param
* @param value
* the value of the property field
* @param defaultValue
* the default value, if no other value is non-null
* @param attribute
* the component attribute being evaluated
* @param component
* the component we are getting the attribute value for
* @param context
* the faces context
* @return value
, the result of evaluating the
* {@link ValueExpression} for attribute
on
* component
, or defaultValue
.
*/
@SuppressWarnings("unchecked")
public static T getAttribute(T value, T defaultValue, String attribute,
UIComponent component, FacesContext context) {
if (value != null) {
return value;
}
ValueExpression ve = component.getValueExpression(attribute);
return Utils.getValue((ve == null) ? null : (T) ve.getValue(context
.getELContext()), defaultValue);
}
/**
* Convenience method. Creates an iterator that returns the clientId of each
* component in components.
*
* @param components
* @param context
* @return
*/
public static Iterator getIdIterator(
final Iterable components, final FacesContext context) {
return new Iterator() {
private Iterator it = components.iterator();
public boolean hasNext() {
return it.hasNext();
}
public String next() {
return it.next().getClientId(context);
}
public void remove() {
throw new UnsupportedOperationException();
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy