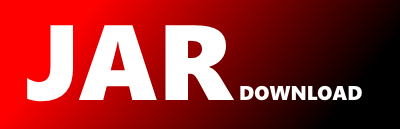
com.jsftoolkit.base.ResourceInfo Maven / Gradle / Ivy
package com.jsftoolkit.base;
import com.jsftoolkit.utils.Utils;
import com.jsftoolkit.utils.serial.XmlAttribute;
import com.jsftoolkit.utils.serial.XmlElement;
/**
* Describes an include in an HTML page, e.g. a script or stylesheet.
*
*
* - id
* - A unique identifier for the resource. It is recommended that it be of
* the form "com.yourcompany.package.ComponentClass.RESOURCENAME_EXT"
* - defaultResource
* - The classpath resource to load if one is not specified by other means.
* - encoding (optional)
* - The encoding to use when reading in the resource (may be overridden).
* - filter (optional)
* - The name of the managed bean that will convert embedded
* resource paths into appropriate remoting URLs. Note that this is the actual
* name, not a value binding.
*
* Each of the defaults, resource, encoding and bean, may be overridden in the
* following order:
*
*
* - If the attribute is set on the component, the set value is used.
*
- Otherwise, if there is a web.xml initialization parameter with the name
* of the attribute, it will be used.
*
- Otherwise, the default value is used.
*
* The attribute name is: the Resource ID for the resource, the Resource ID +
* '.ENCODING' for the encoding, and the Resource ID + '.BEAN' for the bean.
* e.g. specifying
* com.yourcompany.package.ComponentClass.RESOURCENAME_EXT="/com/clientcompany/theResource.ext"
* on the component will override the resource value for that component.
* Specifying it in web.xml will override it for all instances of that
* component.
*
*
* @author noah
*
*/
@XmlElement(tagName = "resource")
public class ResourceInfo {
/**
* Defines the content types. SCRIPT for javascript and STYLE for css.
*
* @author noah
*
*/
public enum Type {
SCRIPT("text/javascript"), STYLE("text/css");
private String contentType;
private Type(String type) {
this.contentType = type;
}
@Override
public String toString() {
return contentType;
}
public static Type decode(String name) {
for (Type type : values()) {
if (type.name().equalsIgnoreCase(name)) {
return type;
}
}
return null;
}
};
private String id;
private String defaultResource;
private String encoding;
private String filter;
private Type type;
public ResourceInfo() {
super();
}
/**
* Any of the parameters except id and defaultResource may be null.
*
* @param id
* the id of the resource, used for loading defaults from web.xml
* and as the attribute name on the component for instance
* override. A good id would be the canonical class name of the
* component + the filename of the resource converted to
* uppercase. e.g. com.jsftoolkit.components.dnd.DragItem.DOJO_JS
* @param defaultResource
* the classpath resource to load be default
* @param type
* the type of the resource
* @param encoding
* @param filter
*/
public ResourceInfo(final String id, final String defaultResource,
final Type type, String encoding, String filter) {
this();
this.id = id;
this.defaultResource = defaultResource;
this.type = type;
this.encoding = encoding;
this.filter = filter;
}
/**
*
* @return the default classpath resource to use
*/
@XmlAttribute
public String getDefaultResource() {
return defaultResource;
}
/**
*
* @return the ID of this resource
*/
@XmlAttribute
public String getId() {
return id;
}
/**
*
* @return the type of this resource.
*/
public Type getResourceType() {
return type;
}
/**
* For Digester, since it can't handle enums.
*
* @return the toString of resource type
*/
@XmlAttribute
public String getType() {
return type == null ? null : type.name();
}
/**
*
* @return the encoding of the resource.
*/
@XmlAttribute
public String getEncoding() {
return encoding;
}
/**
*
* @return the name of the managed bean that should filter the resource.
*/
@XmlAttribute
public String getFilter() {
return filter;
}
public void setDefaultResource(String defaultResource) {
this.defaultResource = defaultResource;
}
public void setEncoding(String encoding) {
this.encoding = encoding;
}
public void setFilter(String filter) {
this.filter = filter;
}
public void setId(String id) {
this.id = id;
}
/**
* For Commons Digester.
*
* @param type
*/
public void setType(String type) {
this.type = Type.decode(type);
}
public void setResourceType(Type type) {
this.type = type;
}
/**
* @see Regenerable#getCodeString()
*/
public CharSequence getCodeString() {
return String.format("new %s(\"%s\",\"%s\",%s,%s,%s)", getClass()
.getSimpleName(), id, defaultResource, type == null ? "null"
: Type.class.getSimpleName() + '.' + type.name(), Utils
.toStringConstant(encoding), Utils.toStringConstant(filter));
}
/**
* Identity is based on id.
*/
@Override
public int hashCode() {
final int PRIME = 31;
int result = 1;
result = PRIME * result + ((id == null) ? 0 : id.hashCode());
return result;
}
/**
* Identity is based on id.
*/
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (getClass() != obj.getClass())
return false;
final ResourceInfo other = (ResourceInfo) obj;
if (id == null) {
if (other.id != null)
return false;
} else if (!id.equals(other.id))
return false;
return true;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy