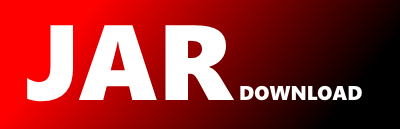
com.jsftoolkit.gen.ConstantsComponentInfoFactory Maven / Gradle / Ivy
package com.jsftoolkit.gen;
import java.beans.IntrospectionException;
import java.io.IOException;
import java.lang.reflect.Field;
import java.lang.reflect.Modifier;
import java.util.Arrays;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Map;
import java.util.Set;
import javax.faces.component.UIComponent;
import javax.xml.parsers.ParserConfigurationException;
import javax.xml.xpath.XPathExpressionException;
import org.xml.sax.SAXException;
import com.jsftoolkit.base.ResourceInfo;
import com.jsftoolkit.base.TagBase;
import com.jsftoolkit.gen.info.ComponentInfo;
import com.jsftoolkit.gen.info.ConfigInfo;
import com.jsftoolkit.gen.info.ConstantInfo;
import com.jsftoolkit.gen.info.PropertyInfo;
import com.jsftoolkit.utils.Utils;
/**
* Factory for {@link ComponentInfo} instances derived from a class of
* constants.
*
* @author noah
*
*/
public class ConstantsComponentInfoFactory {
private static final String REGISTER_RENDERER = "REGISTER_RENDERER";
public static final String PACKAGE = "PACKAGE";
public static final String CLASS_NAME = "CLASS_NAME";
public static final String COMPONENT_TYPE = "COMPONENT_TYPE";
public static final String COMPONENT_FAMILY = "COMPONENT_FAMILY";
public static final String DEFAULT_RENDERER_TYPE = "DEFAULT_RENDERER_TYPE";
public static final String SUPER_CLASS = "SUPER_CLASS";
public static final String ABSTRACT = "ABSTRACT";
public static final String IMPLEMENTS = "IMPLEMENTS";
public static final String IMPORTS = "IMPORTS";
public static final String RENDERER_PACKAGE = "RENDERER_PACKAGE";
public static final String RENDERER_CLASS_NAME = "RENDERER_CLASS_NAME";
public static final String RENDERER_ABSTRACT = "RENDERER_ABSTRACT";
public static final String RENDERER_IMPLEMENTS = "RENDERER_IMPLEMENTS";
public static final String REGISTER_CLASS = "REGISTER_CLASS";
/**
* String[] of names of attributes that should appear on the tag handler but
* do not need a constant or getter/setter pair.
*/
public static final String RENDER_ATTRIBS = "RENDER_ATTRIBS";
public static final String TAG_SUPER = "TAG_SUPER";
public static final String TAG_PACKAGE = "TAG_PACKAGE";
public static final String TAG_CLASS_NAME = "TAG_CLASS_NAME";
public static final String TAG_ABSTRACT = "TAG_ABSTRACT";
public static final String INCLUDES = "INCLUDES";
public static final String TEMPLATE = "TEMPLATE";
public static final String HEAD_TEMPLATE = "HEAD_TEMPLATE";
public static final String TEMPLATE_ENCODING = "TEMPLATE_ENCODING";
public static final String FACES_CONFIG = "FACES_CONFIG";
public static final String TAGLIB_XML = "TAGLIB_XML";
public static final String TLD = "TLD";
public static final int PUBLIC_STATIC_FINAL = Modifier.PUBLIC
| Modifier.STATIC | Modifier.FINAL;
private static final String NAMESPACE = "NAMESPACE";
private static final String SHORT_NAME = "SHORT_NAME";
private static final String TAG_NAME = "TAG_NAME";
public static final String[] IGNORE_CONSTANTS;
static {
Set list = new HashSet();
for (Field field : ConstantsComponentInfoFactory.class.getFields()) {
if ((field.getModifiers() & PUBLIC_STATIC_FINAL) == PUBLIC_STATIC_FINAL) {
list.add(field.getName());
}
}
IGNORE_CONSTANTS = list.toArray(new String[list.size()]);
}
/**
*
* @param spec
* a class containing the above constants
* @return a {@link ComponentInfo} instance derived from the constants in
* spec.
* @throws Exception
*/
public static ComponentInfo parse(final Class> spec) throws Exception {
ComponentInfo info = new ComponentInfo((String) getConstant(PACKAGE,
spec), (String) getConstant(CLASS_NAME, spec),
(String) getConstant(COMPONENT_FAMILY, spec),
(String) getConstant(COMPONENT_TYPE, spec),
(String) getConstant(DEFAULT_RENDERER_TYPE, spec));
Set> imports = new HashSet>();
Map properties = new HashMap();
Set constants = new HashSet();
// find the important constants
for (Field field : spec.getFields()) {
if ((field.getModifiers() & PUBLIC_STATIC_FINAL) == PUBLIC_STATIC_FINAL) {
String name = field.getName();
Object value = field.get(null);
if (value instanceof PropertyInfo) {
PropertyInfo pInfo = (PropertyInfo) value;
Class> c1 = pInfo.getType();
properties.put(name, pInfo);
if (c1 != null && !c1.isPrimitive()) {
imports.add(c1);
}
} else if (value instanceof String) {
constants.add(new ConstantInfo(name, (String) value));
}
}
}
// remove the special constants. they are specified elsewhere
for (String name : IGNORE_CONSTANTS) {
constants.remove(new ConstantInfo(name, null));
}
imports.addAll(Arrays.asList(Utils.getValue((Class>[]) getConstant(
IMPORTS, spec), new Class>[0])));
info.setImports(imports);
info.setConstants(constants);
info.setProperties(properties);
info.setPackage(((String) getConstant(PACKAGE, spec)));
info.setClassName((String) getConstant(CLASS_NAME, spec));
info.setAbstract(Boolean.valueOf(Utils.toString(getConstant(ABSTRACT,
spec))));
info.setSuperClass((Class extends UIComponent>) getConstant(
SUPER_CLASS, spec));
info.setInterfaces(Utils.asSet((Class>[]) getConstant(IMPLEMENTS,
spec)));
info.getTag().setPackage((String) getConstant(TAG_PACKAGE, spec));
info.getTag().setClassName((String) getConstant(TAG_CLASS_NAME, spec));
info.getRenderer().setAttribs(
Utils.asSet((String[]) getConstant(RENDER_ATTRIBS, spec)));
info.getRenderer().setAbstract(
Boolean.valueOf(Utils.toString(getConstant(RENDERER_ABSTRACT,
spec))));
info.getTag().setSuperClass(
(Class extends TagBase>) getConstant(TAG_SUPER, spec));
info.getTag().setAbstract(
Boolean
.valueOf(Utils
.toString(getConstant(TAG_ABSTRACT, spec))));
info.getConfig().setRegisterRenderer(
Utils.toString(getConstant(REGISTER_RENDERER, spec), null));
info.getRenderer().setClassName(
(String) getConstant(RENDERER_CLASS_NAME, spec));
info.getRenderer().setPackage(
(String) getConstant(RENDERER_PACKAGE, spec));
info.getRenderer().setTemplate((String) getConstant(TEMPLATE, spec));
info.getRenderer().setHeadTemplate(
(String) getConstant(HEAD_TEMPLATE, spec));
info.getRenderer().setTemplateEncoding(
(String) getConstant(TEMPLATE_ENCODING, spec));
Object constant = getConstant(INCLUDES, spec);
if (constant instanceof ResourceInfo[]) {
for (ResourceInfo resource : (ResourceInfo[]) constant) {
info.getRenderer().addInclude(resource);
System.out.println("Added include "
+ resource.getDefaultResource());
}
}
info.getConfig().setFacesConfig(
Utils.toString(getConstant(FACES_CONFIG, spec), null));
info.getConfig().setTaglibXml(
Utils.toString(getConstant(TAGLIB_XML, spec), null));
info.getConfig().setTldFile(
Utils.toString(getConstant(TLD, spec), null));
info.getConfig().setNamespace(
Utils.toString(getConstant(NAMESPACE, spec), null));
info.getConfig().setLibraryShortName(
Utils.toString(getConstant(SHORT_NAME, spec), null));
info.getConfig().setTagName(
Utils.toString(getConstant(TAG_NAME, spec), null));
info.getConfig().setRegisterClass(
Utils.toString(getConstant(REGISTER_CLASS, spec), null));
return info;
}
/**
*
* @param name
* @param spec
* @return the value of the constant with the given name
* @throws Exception
*/
private static Object getConstant(String name, Class> spec)
throws Exception {
try {
return spec.getField(name).get(null);
} catch (NoSuchFieldException e) {
return null;
}
}
/**
* Generates the code and updates the configuration files for all of the
* given components, inferring the necessary parameters.
*
* @param specs
* @throws Exception
* @throws ParserConfigurationException
* @throws SAXException
* @throws IOException
* @throws XPathExpressionException
* @throws IntrospectionException
*/
public static void genAndUpdateAll(Class>... specs) throws Exception,
ParserConfigurationException, SAXException, IOException,
XPathExpressionException, IntrospectionException {
genAndUpdateAll(null, specs);
}
public static void genAndUpdateAll(ConfigInfo config, Class>... specs)
throws Exception, ParserConfigurationException, SAXException,
IOException, XPathExpressionException, IntrospectionException {
ComponentInfo[] infos = new ComponentInfo[specs.length];
TemplateComponentGenerator generator = new TemplateComponentGenerator();
int i = 0;
for (Class> spec : specs) {
ComponentInfo info = infos[i++] = ConstantsComponentInfoFactory
.parse(spec);
generator.generate(info);
}
if (i > 0) {
if (config == null) {
config = ConfigInfo.newWithDefaults(infos[0]);
}
ConfigurationUpdater updater = ConfigurationUpdater.getInstance();
updater.updateFacesConfig(updater.getFacesConfig(config
.getFacesConfig()), infos);
updater.updateTaglibXml(updater.getTaglib(config.getNamespace(),
config.getTaglibXml()), infos);
updater.updateTld(updater.getTld(config.getTldFile(), config
.getNamespace(), config.getLibraryShortName()), infos);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy