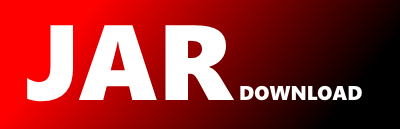
com.jsftoolkit.gen.XmlComponentInfoFactory Maven / Gradle / Ivy
Go to download
The core classes for the JSF Toolkit Component Framework. Includes all
framework base and utility classes as well as component
kick-start/code-generation and registration tools.
Also includes some classes for testing that are reused in other projects.
They cannot be factored out into a separate project because they are
referenced by the tests and they reference this code (circular
dependence).
The newest version!
Please wait ...
© 2015 - 2025 Weber Informatics LLC | Privacy Policy