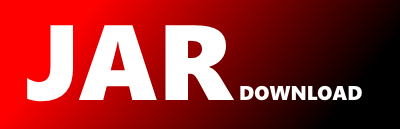
com.jsftoolkit.gen.info.ComponentInfo Maven / Gradle / Ivy
package com.jsftoolkit.gen.info;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Map;
import java.util.Set;
import javax.faces.component.UIComponent;
import javax.faces.component.UIComponentBase;
import javax.faces.render.Renderer;
import javax.faces.webapp.UIComponentELTag;
import com.jsftoolkit.utils.serial.XmlAttribute;
import com.jsftoolkit.utils.serial.XmlCollection;
import com.jsftoolkit.utils.serial.XmlElement;
import com.jsftoolkit.utils.serial.XmlFollow;
/**
* Describes a {@link UIComponent}, it's {@link UIComponentELTag}, and
* possibly its {@link Renderer}. It also contains the necessary information to
* update faces-config.xml, taglib.xml files and .tld files.
*
* Provides sensible defaults. Attempts to set most properties to null will be
* ignored.
*
* @author noah
*/
@XmlElement(tagName = "component")
public class ComponentInfo extends ClassInfo {
private Class extends UIComponent> superClass = UIComponentBase.class;
private Map properties = new HashMap();
private Set constants = new HashSet();
private String rendererType;
private String family;
private String type;
private RendererInfo renderer;
private TagInfo tag;
private ConfigInfo config;
/**
* Convenience constructor.
*
* @param _package
* @param className
* @param family
* @param type
* @param rendererType
*/
public ComponentInfo(String _package, String className, String family,
String type, String rendererType) {
this();
this.setPackage(_package);
this.setClassName(className);
this.rendererType = rendererType;
this.family = family;
this.type = type;
}
/**
* Default constructor. Initializes config, renderer, and tag with new
* instances using the {@link ChildInfo#ChildInfo(ClassInfo)} constructor.
*
*/
public ComponentInfo() {
super();
this.config = new ConfigInfo(this);
this.renderer = new RendererInfo(this);
this.tag = new TagInfo(this);
}
/**
*
* @return the set of extra constants this component should declare. Should
* rarely be needed.
*/
@XmlCollection
public Set getConstants() {
return constants;
}
/**
*
* @return the properties of the component, in a map for convenient lookup
* by name.
*/
@XmlCollection
public Map getProperties() {
return properties;
}
/**
*
* @return the super class of this component
*/
@XmlAttribute
public Class extends UIComponent> getSuperClass() {
return superClass;
}
/**
*
* @return the family of the component
*/
@XmlAttribute
public String getFamily() {
return family;
}
/**
*
* @return the default renderer type of the component
*/
@XmlAttribute
public String getRendererType() {
return rendererType;
}
/**
*
* @return the component type
*/
@XmlAttribute
public String getType() {
return type;
}
public void setType(String type) {
if (type != null) {
this.type = type;
}
}
public void setFamily(String componentFamily) {
if (componentFamily != null) {
this.family = componentFamily;
}
}
public void setRendererType(String defaultRendererType) {
if (defaultRendererType != null) {
this.rendererType = defaultRendererType;
}
}
public void setConstants(Set constants) {
if (constants != null) {
this.constants = constants;
}
}
public void setProperties(Map properties) {
if (properties != null) {
this.properties = properties;
}
}
public void setSuperClass(Class extends UIComponent> superClass) {
if (superClass != null) {
this.superClass = superClass;
}
}
/**
*
* @return information about the configuration files to be updated for this
* component
*/
@XmlFollow
public ConfigInfo getConfig() {
return config;
}
public void setConfig(ConfigInfo config) {
this.config = config;
}
/**
*
* @return information about the component's renderer
*/
@XmlFollow
public RendererInfo getRenderer() {
return renderer;
}
public void setRenderer(RendererInfo renderer) {
this.renderer = renderer;
}
/**
*
* @return information about the component's tag handler
*/
@XmlFollow
public TagInfo getTag() {
return tag;
}
public void setTag(TagInfo tag) {
this.tag = tag;
}
/**
* Adds a constant.
*
* @param o
*/
public void addConstant(ConstantInfo o) {
constants.add(o);
}
/**
* Adds a property.
*
* @param info
*/
public void addProperty(PropertyInfo info) {
properties.put(info.getName(), info);
}
}