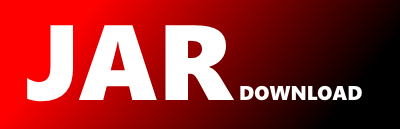
com.jsftoolkit.utils.serial.AnnotatedObjectDomSerializer Maven / Gradle / Ivy
package com.jsftoolkit.utils.serial;
import java.beans.BeanInfo;
import java.beans.IntrospectionException;
import java.beans.Introspector;
import java.beans.PropertyDescriptor;
import java.lang.reflect.Method;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import org.w3c.dom.Node;
import com.jsftoolkit.utils.Utils;
/**
* {@link #toDom(Object, Document, DomSerializerManager)}
*
* @author noah
*
*/
public class AnnotatedObjectDomSerializer implements DomSerializer
© 2015 - 2025 Weber Informatics LLC | Privacy Policy