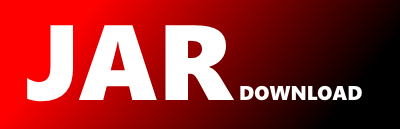
com.jslsolucoes.db.migrate.se.cli.ConsoleMode Maven / Gradle / Ivy
package com.jslsolucoes.db.migrate.se.cli;
import java.io.File;
import java.io.FileReader;
import java.nio.file.Paths;
import java.util.Properties;
import org.apache.commons.cli.CommandLine;
import org.apache.commons.cli.CommandLineParser;
import org.apache.commons.cli.DefaultParser;
import org.apache.commons.cli.HelpFormatter;
import org.apache.commons.cli.Option;
import org.apache.commons.cli.Options;
import org.apache.commons.lang3.StringUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class ConsoleMode {
private String[] args;
private final static Logger logger = LoggerFactory.getLogger(ConsoleMode.class);
private ConsoleMode() {
}
public static ConsoleMode newConsole() {
return new ConsoleMode();
}
public ConsoleMode withArgs(String[] args) {
this.args = args;
return this;
}
public ConsoleArgument build() {
try {
Options options = new Options();
options.addOption(new Option("h", false, "Help"));
options.addOption(new Option("c", true, "Configuration file location"));
options.addOption(new Option("s", true, "Scripts folder location"));
CommandLineParser commandLineParser = new DefaultParser();
CommandLine commandLine = commandLineParser.parse(options, args, true);
ConsoleArgument consoleArgument = new ConsoleArgument();
if (commandLine.hasOption("help")) {
HelpFormatter helpFormatter = new HelpFormatter();
helpFormatter.printHelp("java -jar tjmsprh-rh-clock-sync.jar -conf application.properties", options);
throw new RuntimeException("Help called. Program will exit..");
}
for (Option option : options.getOptions()) {
String arg = option.getOpt();
if (option.hasArg() && StringUtils.isEmpty(commandLine.getOptionValue(arg))) {
logger.error("Argument -{} is required to launch. Use -help to see arguments", arg);
throw new RuntimeException("Argument -"+arg+" is required to launch. Use -help to see arguments");
}
}
consoleArgument.setConfiguration(properties(new File(commandLine.getOptionValue("c"))));
consoleArgument.setScript(Paths.get(commandLine.getOptionValue("s")));
return consoleArgument;
} catch (Exception e) {
throw new RuntimeException(e);
}
}
private Properties properties(File file) {
try (FileReader fileReader = new FileReader(file)) {
Properties properties = new Properties();
properties.load(fileReader);
return properties;
} catch (Exception e) {
throw new RuntimeException(e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy