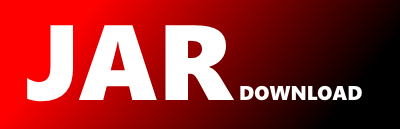
com.jslsolucoes.jax.rs.client.se.api.impl.ServiceUnavailableResponse Maven / Gradle / Ivy
package com.jslsolucoes.jax.rs.client.se.api.impl;
import java.lang.annotation.Annotation;
import java.net.URI;
import java.util.Date;
import java.util.Locale;
import java.util.Map;
import java.util.Set;
import javax.ws.rs.core.EntityTag;
import javax.ws.rs.core.GenericType;
import javax.ws.rs.core.Link;
import javax.ws.rs.core.Link.Builder;
import javax.ws.rs.core.MediaType;
import javax.ws.rs.core.MultivaluedMap;
import javax.ws.rs.core.NewCookie;
import javax.ws.rs.core.Response;
import javax.ws.rs.core.Response.Status.Family;
public class ServiceUnavailableResponse extends Response {
@Override
public int getStatus() {
return 503;
}
@Override
public StatusType getStatusInfo() {
return new StatusType() {
@Override
public int getStatusCode() {
return getStatus();
}
@Override
public String getReasonPhrase() {
return "Request failed after some attempts";
}
@Override
public Family getFamily() {
return null;
}
};
}
@Override
public Object getEntity() {
throw new UnsupportedOperationException("Operation not supported for fallback response");
}
@Override
public T readEntity(Class entityType) {
throw new UnsupportedOperationException("Operation not supported for fallback response");
}
@Override
public T readEntity(GenericType entityType) {
throw new UnsupportedOperationException("Operation not supported for fallback response");
}
@Override
public T readEntity(Class entityType, Annotation[] annotations) {
throw new UnsupportedOperationException("Operation not supported for fallback response");
}
@Override
public T readEntity(GenericType entityType, Annotation[] annotations) {
throw new UnsupportedOperationException("Operation not supported for fallback response");
}
@Override
public boolean hasEntity() {
throw new UnsupportedOperationException("Operation not supported for fallback response");
}
@Override
public boolean bufferEntity() {
throw new UnsupportedOperationException("Operation not supported for fallback response");
}
@Override
public void close() {
}
@Override
public MediaType getMediaType() {
throw new UnsupportedOperationException("Operation not supported for fallback response");
}
@Override
public Locale getLanguage() {
throw new UnsupportedOperationException("Operation not supported for fallback response");
}
@Override
public int getLength() {
throw new UnsupportedOperationException("Operation not supported for fallback response");
}
@Override
public Set getAllowedMethods() {
throw new UnsupportedOperationException("Operation not supported for fallback response");
}
@Override
public Map getCookies() {
throw new UnsupportedOperationException("Operation not supported for fallback response");
}
@Override
public EntityTag getEntityTag() {
throw new UnsupportedOperationException("Operation not supported for fallback response");
}
@Override
public Date getDate() {
throw new UnsupportedOperationException("Operation not supported for fallback response");
}
@Override
public Date getLastModified() {
throw new UnsupportedOperationException("Operation not supported for fallback response");
}
@Override
public URI getLocation() {
throw new UnsupportedOperationException("Operation not supported for fallback response");
}
@Override
public Set getLinks() {
throw new UnsupportedOperationException("Operation not supported for fallback response");
}
@Override
public boolean hasLink(String relation) {
throw new UnsupportedOperationException("Operation not supported for fallback response");
}
@Override
public Link getLink(String relation) {
throw new UnsupportedOperationException("Operation not supported for fallback response");
}
@Override
public Builder getLinkBuilder(String relation) {
throw new UnsupportedOperationException("Operation not supported for fallback response");
}
@Override
public MultivaluedMap getMetadata() {
throw new UnsupportedOperationException("Operation not supported for fallback response");
}
@Override
public MultivaluedMap getStringHeaders() {
throw new UnsupportedOperationException("Operation not supported for fallback response");
}
@Override
public String getHeaderString(String name) {
throw new UnsupportedOperationException("Operation not supported for fallback response");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy