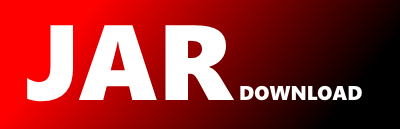
com.jslsolucoes.maven.plugin.resource.ReplaceRegExpResourceMojo Maven / Gradle / Ivy
package com.jslsolucoes.maven.plugin.resource;
import java.io.File;
import java.io.FileInputStream;
import java.util.List;
import java.util.Properties;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import org.apache.commons.io.FileUtils;
import org.apache.commons.lang3.StringUtils;
import org.apache.maven.plugin.AbstractMojo;
import org.apache.maven.plugin.MojoExecutionException;
import org.apache.maven.plugin.MojoFailureException;
import org.apache.maven.plugins.annotations.Mojo;
import org.apache.maven.plugins.annotations.Parameter;
@Mojo(name = "replace")
public class ReplaceRegExpResourceMojo extends AbstractMojo {
@Parameter(property = "skip")
private boolean skip = false;
@Parameter(property = "properties")
private File properties;
@Parameter(property = "replaces", required = true)
private List replaces;
public ReplaceRegExpResourceMojo() {
}
public ReplaceRegExpResourceMojo(boolean skip, File properties, List replaces) {
this.skip = skip;
this.properties = properties;
this.replaces = replaces;
}
@Override
public void execute() throws MojoExecutionException, MojoFailureException {
if (!skip) {
Properties properties = properties();
for (Replace replace : replaces) {
if (!replace.isSkip()) {
replace(replace, properties);
} else {
getLog().info("Skipping replace " + replace.getFile());
}
}
} else {
getLog().info("Skipping replace execution");
}
}
private void replace(Replace replace, Properties properties) {
try {
File file = replace.getFile();
String encoding = replace.getEncoding();
String fileContent = FileUtils.readFileToString(file, encoding);
for (Token token : replace.getTokens()) {
String match = token.getMatch();
String matchReplace = token.getReplace();
String matchReplaceValue = matchReplaceValue(matchReplace, properties);
fileContent = Pattern.compile(match, Pattern.MULTILINE).matcher(fileContent)
.replaceAll(matchReplaceValue);
}
getLog().info("Final file content " + fileContent);
FileUtils.writeStringToFile(file, fileContent, encoding);
getLog().info("File " + file + " was successfully processed");
} catch (Exception e) {
if (replace.getExitOnFail()) {
throw new RuntimeException(e);
} else {
getLog().info("Skipping failure. Message: " + e.getMessage());
}
}
}
private String matchReplaceValue(String matchReplace, Properties properties) {
String matchReplaceValue = StringUtils.isEmpty(matchReplace) ? "" : new String(matchReplace);
Matcher matcher = Pattern.compile("(@([a-zA-z0-9\\.]+))").matcher(matchReplaceValue.replace("\\", ""));
while (matcher.find()) {
String property = matcher.group(1);
String propertyKey = matcher.group(2);
String propertyValue = properties.getProperty(propertyKey);
if (propertyValue == null) {
getLog().error("Property key " + propertyKey + " was not found on env file " + properties);
} else {
matchReplaceValue = matchReplaceValue.replaceAll(property, propertyValue);
}
}
return matchReplaceValue.replaceAll("\\\\", "\\\\\\\\");
}
private Properties properties() {
try {
Properties properties = new Properties();
properties.load(new FileInputStream(this.properties));
return properties;
} catch (Exception e) {
throw new RuntimeException(e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy