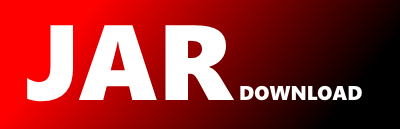
com.jslsolucoes.properties.ee.factory.PropertyValueInjector Maven / Gradle / Ivy
The newest version!
package com.jslsolucoes.properties.ee.factory;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.io.InputStream;
import java.lang.reflect.Field;
import java.nio.file.Files;
import java.nio.file.Paths;
import java.nio.file.StandardOpenOption;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Optional;
import java.util.UUID;
import javax.enterprise.context.Dependent;
import javax.enterprise.inject.Produces;
import javax.enterprise.inject.spi.InjectionPoint;
import javax.inject.Inject;
import org.apache.commons.lang3.StringUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.jslsolucoes.properties.PropertiesBuilder;
import com.jslsolucoes.properties.ee.Properties;
import com.jslsolucoes.properties.ee.PropertyValue;
public class PropertyValueInjector {
private Map cache = new HashMap();
private static Logger logger = LoggerFactory.getLogger(PropertyValueInjector.class);
@Produces
@PropertyValue
@Dependent
public String propertyString(InjectionPoint injectionPoint) {
Class> clazz = injectionPoint.getBean().getBeanClass();
Properties properties = clazz.getAnnotation(Properties.class);
List defaults = defaultsOf(clazz);
PropertyValue propertyValue = injectionPoint.getAnnotated().getAnnotation(PropertyValue.class);
return property(properties, defaults, propertyValue);
}
@Produces
@PropertyValue
@Dependent
public Boolean propertyBoolean(InjectionPoint injectionPoint) {
return Boolean.valueOf(propertyString(injectionPoint));
}
@Produces
@PropertyValue
@Dependent
public Long propertyLong(InjectionPoint injectionPoint) {
return Long.valueOf(propertyString(injectionPoint));
}
@Produces
@PropertyValue
@Dependent
public Integer propertyInteger(InjectionPoint injectionPoint) {
return Integer.valueOf(propertyString(injectionPoint));
}
private String property(Properties properties, List defaults, PropertyValue propertyValue) {
String value = properties(properties, defaults).getProperty(propertyValue.key());
if(StringUtils.isEmpty(value)) {
return propertyValue.defaultValue();
} else {
return value;
}
}
private java.util.Properties properties(Properties properties, List defaults) {
String name = properties.value();
if (!cache.containsKey(name)) {
logger.debug("Creating or loading properties file {}", name);
java.util.Properties props = new java.util.Properties();
try {
props.load(findOrBuild(name, properties(defaults)));
} catch (IOException ioException) {
logger.error("Could not load application properties {} ", name, ioException);
}
cache.put(name, PropertiesBuilder.newBuilder().withProperties(props).build());
} else {
logger.debug("Properties file {} already load ", name);
}
return cache.get(name);
}
private List defaultsOf(Class> clazz) {
List properties = new ArrayList();
for (Field field : clazz.getDeclaredFields()) {
if(field.isAnnotationPresent(PropertyValue.class)) {
PropertyValue propertyValue = field.getAnnotation(PropertyValue.class);
properties.add(new Property(propertyValue.key(), propertyValue.defaultValue()));
} else if(field.isAnnotationPresent(Inject.class)) {
properties.addAll(defaultsOf(field.getType()));
}
}
return properties;
}
private InputStream findOrBuild(String resource, Map properties) {
Optional inputStream = classpath("/" + resource);
if (!inputStream.isPresent()) {
inputStream = file(System.getProperty(resource, build(properties)));
}
return inputStream.get();
}
private String build(Map properties) {
try {
String uuid = UUID.randomUUID().toString() + ".properties";
logger.debug("Creating temporary file " + uuid + " with default properties");
return Files
.write(Paths.get(System.getProperty("java.io.tmpdir"), uuid),
content(properties).getBytes("ISO-8859-1"), StandardOpenOption.CREATE_NEW)
.toFile().getAbsolutePath();
} catch (IOException e) {
throw new RuntimeException(e);
}
}
private String content(Map properties) {
StringBuilder content = new StringBuilder();
for (Entry entry : properties.entrySet()) {
content.append(entry.getKey() + "=" + entry.getValue() + "\n");
}
return content.toString();
}
private Map properties(List properties) {
Map defaults = new LinkedHashMap<>();
for (Property property : properties) {
defaults.put(property.getKey(), System.getProperty(property.getKey(), property.getDefaultValue()));
}
return defaults;
}
private Optional file(String path) {
try {
logger.debug("Loading properties from file: " + path);
return Optional.of(new FileInputStream(new File(path)));
} catch (FileNotFoundException e) {
throw new RuntimeException(e);
}
}
private Optional classpath(String path) {
logger.debug("Loading properties from classpath: " + path);
return Optional.ofNullable(getClass().getResourceAsStream(path));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy