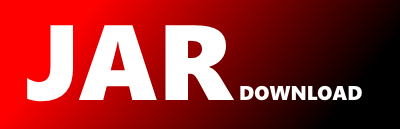
com.jslsolucoes.spring.converter.LocalDateTimeConverter Maven / Gradle / Ivy
package com.jslsolucoes.spring.converter;
import java.time.LocalDateTime;
import java.time.format.DateTimeFormatter;
import java.time.format.DateTimeParseException;
import java.util.Arrays;
import java.util.List;
import org.apache.commons.lang3.StringUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.core.convert.converter.Converter;
public class LocalDateTimeConverter implements Converter {
private static final Logger logger = LoggerFactory.getLogger(LocalDateTimeConverter.class);
@Override
public LocalDateTime convert(String value) {
if (StringUtils.isEmpty(value))
return null;
String valueForMatch = normalize(value);
List patterns = Arrays.asList("yyyy-MM-dd HH:mm:ss", "yyyy-MM-dd HH:mm", "yyyy-MM-dd HH",
"dd/MM/yyyy HH:mm:ss", "dd/MM/yyyy HH:mm", "dd/MM/yyyy HH");
for (String pattern : patterns) {
try {
return LocalDateTime.parse(valueForMatch, DateTimeFormatter.ofPattern(pattern));
} catch (DateTimeParseException e) {
logger.debug("pattern {} does not match with value {}", pattern, value);
}
}
throw new RuntimeException("Could not parse " + value + " to java.time.LocalDateTime");
}
private String normalize(String value) {
if (!value.matches("^[0-9]{2,4}(-|\\/)[0-9]{2}(-|\\/)[0-9]{2,4} [0-9]{2}(:[0-9]{2})?(:[0-9]{2})?$")) {
return value.concat(" 00:00:00");
}
return value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy