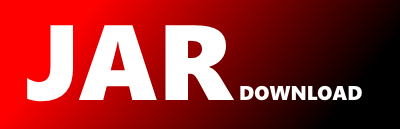
com.jwebmp.plugins.datatable.DataTablePageConfigurator Maven / Gradle / Ivy
Show all versions of jwebmp-data-tables Show documentation
/*
* Copyright (C) 2017 Marc Magon
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
package com.jwebmp.plugins.datatable;
import com.jwebmp.core.Page;
import com.jwebmp.core.base.angular.AngularPageConfigurator;
import com.jwebmp.core.base.references.CSSReference;
import com.jwebmp.core.base.references.JavascriptReference;
import com.jwebmp.core.base.servlets.enumarations.RequirementsPriority;
import com.jwebmp.core.plugins.PluginInformation;
import com.jwebmp.core.plugins.jquery.JQueryPageConfigurator;
import com.jwebmp.core.services.IPageConfigurator;
import com.jwebmp.plugins.datatable.enumerations.DataTablePlugins;
import com.jwebmp.plugins.datatable.enumerations.DataTableThemes;
import com.jwebmp.plugins.datatable.enumerations.DataTablesSortables;
import javax.validation.constraints.NotNull;
import java.util.EnumSet;
import java.util.LinkedHashSet;
import java.util.Set;
import static com.jwebmp.core.utilities.StaticStrings.*;
/**
* @author GedMarc
*/
@PluginInformation(pluginName = "Data Tables",
pluginUniqueName = "data-tables",
pluginDescription = "DataTables is a plug-in for the " +
"jQuery Javascript library. " +
"It is a highly flexible " +
"tool, based upon the " +
"foundations of progressive " +
"enhancement, and will add advanced interaction controls to any HTML table .",
pluginVersion = "1.10.16",
pluginDependancyUniqueIDs = "jquery",
pluginCategories = "jquery,datatables, tables, ui, " + "web, framework",
pluginSubtitle = "DataTables is very simple to use as a jQuery plug-in with a huge range of customisable option",
pluginGitUrl = "https://github.com/GedMarc/JWebSwing-DataTablesPlugin",
pluginSourceUrl = "https://datatables" + "" + ".net/download/index",
pluginWikiUrl = "https://github.com/GedMarc/JWebSwing-DataTablesPlugin/wiki",
pluginOriginalHomepage = "https://www.datatables.net/",
pluginDownloadUrl = "https://sourceforge.net/projects/jwebswing/files/plugins/DataTablesPlugin.jar/download",
pluginIconUrl = "bower_components/datatables/icon.jpg",
pluginIconImageUrl = "bower_components/datatables/jqdatatables_logo.png",
pluginLastUpdatedDate = "2017/09/29")
public class DataTablePageConfigurator
implements IPageConfigurator
{
private static final String DataTablesOperatorString = "dataTables.";
private static final String DataTablesNameString = "DataTables";
private static final String BowerComponentsString = "bower_components/";
private static final String BowerComponentDataTablesString = "datatables.net-";
private static final String CssString = "/css/";
private static final String JsMinString = ".min.js";
private static final String CssMinString = ".min.css";
private static final long serialVersionUID = 1L;
/**
* If this configurator is enabled
*/
private static boolean enabled = true;
private static EnumSet themes;
private static Set plugins;
private static Set sortables;
private static Set extensions;
/**
* Method isEnabled returns the enabled of this AngularAnimatedChangePageConfigurator object.
*
* If this configurator is enabled
*
* @return the enabled (type boolean) of this AngularAnimatedChangePageConfigurator object.
*/
public static boolean isEnabled()
{
return DataTablePageConfigurator.enabled;
}
/**
* Method setEnabled sets the enabled of this AngularAnimatedChangePageConfigurator object.
*
* If this configurator is enabled
*
* @param mustEnable
* the enabled of this AngularAnimatedChangePageConfigurator object.
*/
public static void setEnabled(boolean mustEnable)
{
DataTablePageConfigurator.enabled = mustEnable;
}
/**
* Switches the theme used for the data table
*
* @param theme
*/
public static void switchTheme(DataTableThemes theme)
{
DataTablePageConfigurator.getThemes()
.clear();
DataTablePageConfigurator.getThemes()
.add(theme);
}
/**
* Gets the list of themes to be rendered with the page
*
* @return
*/
public static Set getThemes()
{
if (DataTablePageConfigurator.themes == null)
{
DataTablePageConfigurator.themes = EnumSet.of(DataTableThemes.DataTables);
}
return DataTablePageConfigurator.themes;
}
/**
* Configure buttons JS References
*/
public static void configureButtons()
{
DataTablePageConfigurator.getExtensions()
.add(DataTableReferencePool.Buttons.getJavaScriptReference());
DataTablePageConfigurator.getExtensions()
.add(DataTableReferencePool.JSZip.getJavaScriptReference());
DataTablePageConfigurator.getExtensions()
.add(DataTableReferencePool.PDFMake.getJavaScriptReference());
DataTablePageConfigurator.getExtensions()
.add(DataTableReferencePool.PDFMakeVFSFonts.getJavaScriptReference());
DataTablePageConfigurator.getExtensions()
.add(DataTableReferencePool.ButtonsColVis.getJavaScriptReference());
DataTablePageConfigurator.getExtensions()
.add(DataTableReferencePool.ButtonsHtml.getJavaScriptReference());
DataTablePageConfigurator.getExtensions()
.add(DataTableReferencePool.ButtonsPrint.getJavaScriptReference());
}
/**
* Any additional JavaScript references to apply
*
* Usually with getOptions().getButtons() to addon the required buttons javascripts
*
* @return
*/
public static Set getExtensions()
{
if (DataTablePageConfigurator.extensions == null)
{
DataTablePageConfigurator.extensions = new LinkedHashSet<>();
}
return DataTablePageConfigurator.extensions;
}
@NotNull
@Override
public Page configure(Page page)
{
if (!page.isConfigured())
{
JQueryPageConfigurator.setRequired(true);
AngularPageConfigurator.setRequired(true);
page.getBody()
.addJavaScriptReference(DataTableReferencePool.JQueryDataTables.getJavaScriptReference());
for (DataTableThemes theme : DataTablePageConfigurator.getThemes())
{
String themeBasePath = "bower_components/datatables.net-" + theme.getData() + "/js/dataTables." + theme.getFilename();
String themeBaseCssPath = "bower_components/datatables.net-" + theme.getData() + DataTablePageConfigurator.CssString;
if (theme.getFilenameOverride() == null || theme.getFilenameOverride()
.isEmpty())
{
themeBaseCssPath += DataTablePageConfigurator.DataTablesOperatorString + theme.getFilename();
}
else
{
themeBaseCssPath += theme.getFilenameOverride();
}
if (!themeBasePath.contains("dataTables.dataTables"))
{
page.getBody()
.addJavaScriptReference(new JavascriptReference(DataTablePageConfigurator.DataTablesNameString + "-" + theme.toString(), 1.1016, themeBasePath +
DataTablePageConfigurator.JsMinString,
16).setPriority(
RequirementsPriority.Third));
}
page.getBody()
.addCssReference(new CSSReference(DataTablePageConfigurator.DataTablesNameString + theme.toString(), 1.1016, themeBaseCssPath +
DataTablePageConfigurator.CssMinString));
configurePlugins(page, theme);
}
DataTablePageConfigurator.getExtensions()
.forEach(a -> page.getBody()
.addJavaScriptReference(a));
}
return page;
}
@Override
public boolean enabled()
{
return DataTablePageConfigurator.enabled;
}
/**
* Configures all the page plugins with the theme
*
* @param page
* @param theme
*/
private void configurePlugins(Page page, DataTableThemes theme)
{
int sortOrder = 16;
for (DataTablePlugins plugin : DataTablePageConfigurator.getPlugins())
{
String jsPath = null;
if (theme == DataTableThemes.DataTables)
{
jsPath = DataTablePageConfigurator.BowerComponentsString +
DataTablePageConfigurator.BowerComponentDataTablesString + plugin.getFilename() + "/js/" + (plugin.isPlugin()
? DataTablePageConfigurator.DataTablesOperatorString +
plugin.getFilename() +
DataTablePageConfigurator.JsMinString
: STRING_EMPTY);
}
else
{
jsPath = DataTablePageConfigurator.BowerComponentsString +
DataTablePageConfigurator.BowerComponentDataTablesString + plugin.getFilename() + STRING_DASH + theme.getData() + "/js/" + (plugin.isPlugin()
? plugin.getFilename() +
"." +
theme.getFilename() +
DataTablePageConfigurator.JsMinString
: STRING_EMPTY);
}
if (theme == DataTableThemes.DataTables)
{
page.getBody()
.addJavaScriptReference(
new JavascriptReference(DataTablePageConfigurator.DataTablesNameString + theme.getData() + plugin.getFilename(), 1.0, jsPath, sortOrder++).setPriority(
RequirementsPriority.Fourth));
}
else
{
page.getBody()
.addJavaScriptReference(
new JavascriptReference(DataTablePageConfigurator.DataTablesNameString + theme.getData() + plugin.getFilename(), 1.0, jsPath, sortOrder++).setPriority(
RequirementsPriority.DontCare));
}
if (plugin.isCss())
{
String cssPath = null;
if (theme == DataTableThemes.DataTables)
{
cssPath = DataTablePageConfigurator.BowerComponentsString +
DataTablePageConfigurator.BowerComponentDataTablesString +
plugin.getFilename() +
STRING_DASH +
theme.getData() +
DataTablePageConfigurator.CssString +
plugin.getFilename() +
STRING_DOT +
(
plugin.isPlugin()
? DataTablePageConfigurator.DataTablesOperatorString + DataTablePageConfigurator.CssMinString
: STRING_EMPTY);
}
else
{
cssPath = DataTablePageConfigurator.BowerComponentsString +
DataTablePageConfigurator.BowerComponentDataTablesString +
plugin.getFilename() +
STRING_DASH +
theme.getData() +
DataTablePageConfigurator.CssString +
plugin.getFilename() +
STRING_DOT +
theme.getFilename() +
DataTablePageConfigurator.CssMinString;
}
page.getBody()
.addCssReference(new CSSReference(DataTablePageConfigurator.DataTablesNameString + theme.getData() + plugin.getFilename(), 1.0, cssPath.replace("..", ".")));
}
DataTablePageConfigurator.getSortables()
.forEach(a -> page.getBody()
.addJavaScriptReference(a.getReference()));
}
}
/**
* Returns the applied plugins
*
* @return
*/
public static Set getPlugins()
{
if (DataTablePageConfigurator.plugins == null)
{
DataTablePageConfigurator.plugins = new LinkedHashSet<>();
}
return DataTablePageConfigurator.plugins;
}
/**
* Returns the sortables to apply
*
* @return
*/
public static Set getSortables()
{
if (DataTablePageConfigurator.sortables == null)
{
DataTablePageConfigurator.sortables = new LinkedHashSet<>();
}
return DataTablePageConfigurator.sortables;
}
}