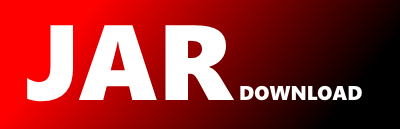
com.jwebmp.plugins.jqplot.options.series.JQPlotSeriesPieOptions Maven / Gradle / Ivy
Show all versions of jwebmp-jqplot Show documentation
/*
* Copyright (C) 2017 GedMarc
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
package com.jwebmp.plugins.jqplot.options.series;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.jwebmp.core.htmlbuilder.javascript.JavaScriptPart;
import com.jwebmp.plugins.jqplot.JQPlotGraph;
import com.jwebmp.plugins.jqplot.parts.interfaces.JQPlotSeriesRenderer;
import jakarta.validation.constraints.NotNull;
import java.util.List;
/**
* The properties for a line graph
*
* @author GedMarc
* @version 1.0
* @since 09 Aug 2015
*/
public class JQPlotSeriesPieOptions>
extends JavaScriptPart
implements JQPlotSeriesRenderer
{
/**
* The graph that this is linked to
*/
@JsonIgnore
private JQPlotGraph linkedGraph;
/**
* Outer diameter of the pie, auto computed by default
*/
private Integer diameter;//
/**
* padding between the pie and plot edges, legend, etc.
*/
private Double padding;
/**
* angular spacing between pie slices in degrees.
*/
private Integer sliceMargin;
/**
* true or false, whether to fil the slices.
*/
private Boolean fill;
/**
* offset of the shadow from the slice and offset of each succesive stroke of the shadow from the last.
*/
private Integer shadowOffset;
/**
* transparency of the shadow (0 = transparent, 1 = opaque)
*/
private Double shadowAlpha;
/**
* number of strokes to apply to the shadow, each stroke offset shadowOffset from the last.
*/
private Integer shadowDepth;
/**
* True to highlight slice when moused over.
*/
private Boolean highlightMouseOver;
/**
* True to highlight when a mouse button is pressed over a slice.
*/
private Boolean highlightMouseDown;
/**
* an array of colors to use when highlighting a slice.
*/
private List highlightColors;
/**
* Either ‘label’, ‘value’, ‘percent’ or an array of labels to place on the pie slices.
*/
private String dataLabels;
/**
* true to show data labels on slices.
*/
private Boolean showDataLabels;
/**
* Format string for data labels.
*/
private String dataLabelFormatString;
/**
* Threshhold in percentage (0-100) of pie area, below which no label will be displayed.
*/
private Integer dataLabelThreshold;
/**
* A Multiplier (0-1) of the pie radius which controls position of label on slice.
*/
private Double dataLabelPositionFactor;
/**
* Number of pixels to slide the label away from (+) or toward (-) the center of the pie.
*/
private Integer dataLabelNudge;
/**
* True to center the data label at its position.
*/
private Boolean dataLabelCenterOn;
/**
* Angle to start drawing pie in degrees.
*/
private Integer startAngle;
/**
* Array for whether the pie chart slice for a data element should be displayed.
*/
private List showSlice;
/**
* Constructs a new Line Options for the given graph
*
* @param linkedGraph
*/
@SuppressWarnings("unchecked")
public JQPlotSeriesPieOptions(JQPlotGraph linkedGraph)
{
this.linkedGraph = linkedGraph;
}
/**
* Gets the graph associated with this options
*
* @return
*/
public JQPlotGraph getLinkedGraph()
{
return linkedGraph;
}
/**
* Sets the graph that is linked to this options
*
* @param linkedGraph
*/
@SuppressWarnings("unchecked")
@NotNull
public J setLinkedGraph(JQPlotGraph linkedGraph)
{
this.linkedGraph = linkedGraph;
return (J) this;
}
/**
* Sets the series render to bubble
*
* @return
*/
@Override
public String getRenderer()
{
return "$.jqplot.PieRenderer";
}
/**
* Outer diameter of the pie, auto computed by default
*
* @return
*/
public Integer getDiameter()
{
return diameter;
}
/**
* Outer diameter of the pie, auto computed by default
*
*
*/
@SuppressWarnings("unchecked")
@NotNull
public J setDiameter(Integer diameter)
{
this.diameter = diameter;
return (J) this;
}
/**
* padding between the pie and plot edges, legend, etc.
*
* @return
*/
public Double getPadding()
{
return padding;
}
/**
* padding between the pie and plot edges, legend, etc.
*
*/
@SuppressWarnings("unchecked")
@NotNull
public J setPadding(Double padding)
{
this.padding = padding;
return (J) this;
}
/**
* angular spacing between pie slices in degrees.
*
* @return
*/
public Integer getSliceMargin()
{
return sliceMargin;
}
/**
* angular spacing between pie slices in degrees.
*
*/
@SuppressWarnings("unchecked")
@NotNull
public J setSliceMargin(Integer sliceMargin)
{
this.sliceMargin = sliceMargin;
return (J) this;
}
/**
* true or false, whether to fil the slices.
*
* @return
*/
public Boolean getFill()
{
return fill;
}
/**
* true or false, whether to fil the slices.
*
*/
@SuppressWarnings("unchecked")
@NotNull
public J setFill(Boolean fill)
{
this.fill = fill;
return (J) this;
}
/**
* offset of the shadow from the slice and offset of each succesive stroke of the shadow from the last.
*
* @return
*/
public Integer getShadowOffset()
{
return shadowOffset;
}
/**
* offset of the shadow from the slice and offset of each succesive stroke of the shadow from the last.
*
*/
@SuppressWarnings("unchecked")
@NotNull
public J setShadowOffset(Integer shadowOffset)
{
this.shadowOffset = shadowOffset;
return (J) this;
}
/**
* transparency of the shadow (0 = transparent, 1 = opaque)
*
* @return
*/
public Double getShadowAlpha()
{
return shadowAlpha;
}
/**
* transparency of the shadow (0 = transparent, 1 = opaque)
*
*
*/
@SuppressWarnings("unchecked")
@NotNull
public J setShadowAlpha(Double shadowAlpha)
{
this.shadowAlpha = shadowAlpha;
return (J) this;
}
/**
* number of strokes to apply to the shadow, each stroke offset shadowOffset from the last.
*
* @return
*/
public Integer getShadowDepth()
{
return shadowDepth;
}
/**
* number of strokes to apply to the shadow, each stroke offset shadowOffset from the last.
*
*/
@SuppressWarnings("unchecked")
@NotNull
public J setShadowDepth(Integer shadowDepth)
{
this.shadowDepth = shadowDepth;
return (J) this;
}
/**
* True to highlight slice when moused over.
*
* @return
*/
public Boolean getHighlightMouseOver()
{
return highlightMouseOver;
}
/**
* True to highlight slice when moused over.
*
*/
@SuppressWarnings("unchecked")
@NotNull
public J setHighlightMouseOver(Boolean highlightMouseOver)
{
this.highlightMouseOver = highlightMouseOver;
return (J) this;
}
/**
* True to highlight when a mouse button is pressed over a slice.
*
* @return
*/
public Boolean getHighlightMouseDown()
{
return highlightMouseDown;
}
/**
* True to highlight when a mouse button is pressed over a slice.
*
*/
@SuppressWarnings("unchecked")
@NotNull
public J setHighlightMouseDown(Boolean highlightMouseDown)
{
this.highlightMouseDown = highlightMouseDown;
return (J) this;
}
/**
* an array of colors to use when highlighting a slice.
*
* @return
*/
public List getHighlightColors()
{
return highlightColors;
}
/**
* an array of colors to use when highlighting a slice.
*
*/
@SuppressWarnings("unchecked")
@NotNull
public J setHighlightColors(List highlightColors)
{
this.highlightColors = highlightColors;
return (J) this;
}
/**
* Either ‘label’, ‘value’, ‘percent’ or an array of labels to place on the pie slices.
*
* @return
*/
public String getDataLabels()
{
return dataLabels;
}
/**
* Either ‘label’, ‘value’, ‘percent’ or an array of labels to place on the pie slices.
*
*/
@SuppressWarnings("unchecked")
@NotNull
public J setDataLabels(String dataLabels)
{
this.dataLabels = dataLabels;
return (J) this;
}
/**
* true to show data labels on slices
*
* @return
*/
public Boolean getShowDataLabels()
{
return showDataLabels;
}
/**
* true to show data labels on slices
*
*/
@SuppressWarnings("unchecked")
@NotNull
public J setShowDataLabels(Boolean showDataLabels)
{
this.showDataLabels = showDataLabels;
return (J) this;
}
/**
* Format string for data labels. If none, ‘%s’ is used for “label? and for arrays, ‘%d’ for value and ‘%d%%’ for percentage.
*
* @return
*/
public String getDataLabelFormatString()
{
return dataLabelFormatString;
}
/**
* Format string for data labels. If none, ‘%s’ is used for “label? and for arrays, ‘%d’ for value and ‘%d%%’ for percentage.
*
*/
@SuppressWarnings("unchecked")
@NotNull
public J setDataLabelFormatString(String dataLabelFormatString)
{
this.dataLabelFormatString = dataLabelFormatString;
return (J) this;
}
/**
* Threshhold in percentage (0-100) of pie area, below which no label will be displayed.
*
* @return
*/
public Integer getDataLabelThreshold()
{
return dataLabelThreshold;
}
/**
* Threshhold in percentage (0-100) of pie area, below which no label will be displayed.
*
*/
@SuppressWarnings("unchecked")
@NotNull
public J setDataLabelThreshold(Integer dataLabelThreshold)
{
this.dataLabelThreshold = dataLabelThreshold;
return (J) this;
}
/**
* A Multiplier (0-1) of the pie radius which controls position of label on slice.
*
* @return
*/
public Double getDataLabelPositionFactor()
{
return dataLabelPositionFactor;
}
/**
* A Multiplier (0-1) of the pie radius which controls position of label on slice.
*
*/
@SuppressWarnings("unchecked")
@NotNull
public J setDataLabelPositionFactor(Double dataLabelPositionFactor)
{
this.dataLabelPositionFactor = dataLabelPositionFactor;
return (J) this;
}
/**
* Number of pixels to slide the label away from (+) or toward (-) the center of the pie.
*
* @return
*/
public Integer getDataLabelNudge()
{
return dataLabelNudge;
}
/**
* Number of pixels to slide the label away from (+) or toward (-) the center of the pie.
*
*/
@SuppressWarnings("unchecked")
@NotNull
public J setDataLabelNudge(Integer dataLabelNudge)
{
this.dataLabelNudge = dataLabelNudge;
return (J) this;
}
/**
* True to center the data label at its position.
*
* @return
*/
public Boolean getDataLabelCenterOn()
{
return dataLabelCenterOn;
}
/**
* True to center the data label at its position.
*
*/
@SuppressWarnings("unchecked")
@NotNull
public J setDataLabelCenterOn(Boolean dataLabelCenterOn)
{
this.dataLabelCenterOn = dataLabelCenterOn;
return (J) this;
}
/**
* Angle to start drawing pie in degrees.
*
* @return
*/
public Integer getStartAngle()
{
return startAngle;
}
/**
* Angle to start drawing pie in degrees.
*
*/
@SuppressWarnings("unchecked")
@NotNull
public J setStartAngle(Integer startAngle)
{
this.startAngle = startAngle;
return (J) this;
}
/**
* Array for whether the pie chart slice for a data element should be displayed.
*
* @return
*/
public List getShowSlice()
{
return showSlice;
}
/**
* Array for whether the pie chart slice for a data element should be displayed.
*
*/
@SuppressWarnings("unchecked")
@NotNull
public J setShowSlice(List showSlice)
{
this.showSlice = showSlice;
return (J) this;
}
}