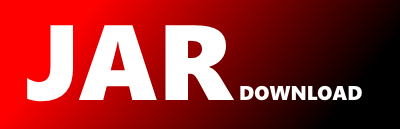
net.sf.uadetector.UserAgentStringParser Maven / Gradle / Ivy
Show all versions of uadetector-core Show documentation
/*******************************************************************************
* Copyright 2012 André Rouél
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
******************************************************************************/
package net.sf.uadetector;
/**
* Basic interface for user agent string parsers.
*
* @author André Rouél
*/
public interface UserAgentStringParser
{
/**
* Returns the current version information of the used UAS data.
*
*
* The version will be set by the UAS data provider (http://user-agent-string.info/) and the version should
* look like this:
*
* format: YYYYMMDD-counter
(counter
is two digits long)
* example: 20120931-02
*
* @return version of the current UAS data
*/
String getDataVersion();
/**
* Detects informations about a network client based on a user agent string.
*
* Typically user agent string will be read by an instance of {@code HttpServletRequest}. With the method
* {@code getHeader("User-Agent")} you can get direct access to this string.
*
* @param userAgent
* user agent string
*
* @return the detected information of an user agent
*/
ReadableUserAgent parse(String userAgent);
/**
* In environments where the JVM will never shut down while reinstalling UADetector, it is necessary to manually
* shutdown running threads of UserAgentStringParser
s with updating functionality like
* UADetectorServiceFactory.getCachingAndUpdatingParser()
or
* UADetectorServiceFactory.getOnlineUpdatingParser()
.
*
* For example, if a web application with UADetector will be re-deployed within an Apache Tomcat you must
* shutdown your self-created or via UADetectorServiceFactory
retrieved updating
* UserAgentStringParser
otherwise more and more threads will be registered.
*
* An implementation of UserAgentStringParser
has updating functionality if it works with a
* {@link net.sf.uadetector.datastore.RefreshableDataStore}.
*
* If you call shutdown on a non-updating UserAgentStringParser
implementation nothing will happen.
*
* A number of Dependency Injection containers support the annotation PreDestroy which is be useful for
* indicating methods that should be called when the container is shutting down. This annotation is available by
* default in Java SE 7 and can be made available through the external library jsr250-api-1.0.jar for earlier
* versions of Java.
*
* We recommend to annotate an implementation of {@link #shutdown()} with PreDestroy to inform a container
* (for example Spring Framework) to trigger the shutdown method automatically by convention during the
* shutdown lifecycle. This saves developers to call explicitly the shutdown method.
*
* To shutdown all managed {@code ExecutorService} by UADetector at once, you can call also
* {@link net.sf.uadetector.internal.util.ExecutorServices#shutdownAll()}.
*/
void shutdown();
}