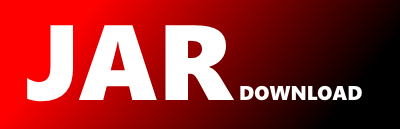
com.kaltura.client.utils.GsonParser Maven / Gradle / Ivy
package com.kaltura.client.utils;
import com.google.gson.Gson;
import com.google.gson.JsonArray;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonParser;
import com.google.gson.JsonPrimitive;
import com.google.gson.JsonSyntaxException;
import com.kaltura.client.types.APIException;
import com.kaltura.client.types.APIException.FailureStep;
import com.kaltura.client.types.ListResponse;
import java.lang.reflect.Constructor;
import java.lang.reflect.InvocationTargetException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* Created by tehilarozin on 24/07/2016.
*/
public class GsonParser {
private static final String ResultKey = "result";
private static final String ObjectTypeKey = "objectType";
private static Gson gson = new Gson();
@SuppressWarnings("unchecked")
public static Class getObjectClass(String objectType, Class defaultClass) {
String className = "com.kaltura.client.types." + objectType.replaceAll("^Kaltura", "");
try {
return (Class) Class.forName(className);
} catch (ClassNotFoundException e) {
return defaultClass;
}
}
public static T parseObject(String result, Class clz) throws APIException {
JsonParser jsonParser = new JsonParser();
JsonElement jsonElement;
try{
jsonElement = jsonParser.parse(result);
}
catch(JsonSyntaxException | IllegalStateException e) {
throw new APIException(FailureStep.OnResponse, "Invalid JSON response: " + result);
}
if(jsonElement.isJsonObject()) {
JsonObject jsonObject = jsonElement.getAsJsonObject();
if(jsonObject.get(ResultKey) != null && jsonObject.get(ObjectTypeKey) == null) {
jsonElement = jsonObject.get(ResultKey);
if (!jsonElement.isJsonPrimitive()) {
jsonObject = jsonElement.getAsJsonObject();
}
}
if(jsonObject.get("error") != null && jsonObject.get(ObjectTypeKey) == null) {
jsonElement = jsonObject.getAsJsonObject("error");
}
}
return parseObject(jsonElement, clz);
}
@SuppressWarnings("unchecked")
public static T parseObject(JsonElement jsonElement, Class clz) throws APIException {
if(jsonElement.isJsonNull()) {
return null;
}
if(jsonElement.isJsonPrimitive()) {
if(clz == String.class) {
return (T)jsonElement.getAsString();
}
if(clz == Integer.class) {
return (T)(Integer)jsonElement.getAsInt();
}
if(clz == Long.class) {
return (T)(Long)jsonElement.getAsLong();
}
if(clz == Boolean.class) {
return (T)(Boolean)jsonElement.getAsBoolean();
}
if(clz == Double.class) {
return (T)(Double)jsonElement.getAsDouble();
}
return (T) (Object) gson.fromJson(jsonElement, Object.class);
}
if(jsonElement.isJsonArray()) {
return (T) parseArray(jsonElement.getAsJsonArray(), clz);
}
return parseObject(jsonElement.getAsJsonObject(), clz);
}
public static T parseObject(JsonObject jsonObject, Class clz) throws APIException {
if(jsonObject == null)
{
return null;
}
JsonPrimitive objectTypeElement = jsonObject.getAsJsonPrimitive(ObjectTypeKey);
if(objectTypeElement == null && jsonObject.has("error")) {
throw parseException(jsonObject.getAsJsonObject("error"));
}
else if(objectTypeElement != null) {
String objectType = objectTypeElement.getAsString();
if(objectType.equals("KalturaAPIException")) {
throw parseException(jsonObject);
}
clz = getObjectClass(objectType, clz);
}
if(clz == Void.class) {
return null;
}
try {
Constructor constructor = clz.getConstructor(JsonObject.class);
return constructor.newInstance(jsonObject);
} catch (NoSuchMethodException | SecurityException | InstantiationException |
IllegalAccessException | IllegalArgumentException | InvocationTargetException |
ClassCastException | IllegalStateException e) {
throw new APIException(FailureStep.OnResponse, e);
}
}
public static List> parseArray(String result, Class>[] types) throws APIException {
JsonParser jsonParser = new JsonParser();
JsonElement jsonElement;
try{
jsonElement = jsonParser.parse(result);
}
catch(JsonSyntaxException | IllegalStateException e) {
throw new APIException(FailureStep.OnResponse, "Invalid JSON response: " + result);
}
if(jsonElement.isJsonObject()) {
JsonObject jsonObject = jsonElement.getAsJsonObject();
if(jsonObject.get(ResultKey) != null && jsonObject.get(ObjectTypeKey) == null) {
jsonElement = jsonObject.get(ResultKey);
}
if(jsonObject.get("error") != null && jsonObject.get(ObjectTypeKey) == null) {
jsonElement = jsonObject.getAsJsonObject("error");
}
}
if(jsonElement.isJsonObject()) {
JsonObject jsonObject = jsonElement.getAsJsonObject();
String objectType = jsonObject.getAsJsonPrimitive(ObjectTypeKey).getAsString();
if(objectType.equals("KalturaAPIException")) {
throw parseException(jsonObject);
}
}
else if(jsonElement.isJsonArray()) {
return parseArray(jsonElement.getAsJsonArray(), types);
}
throw new APIException(FailureStep.OnResponse, "Invalid JSON response type, expected array: " + result);
}
public static List> parseArray(JsonArray jsonArray, Class>[] types) throws APIException {
if(jsonArray == null)
{
return null;
}
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy