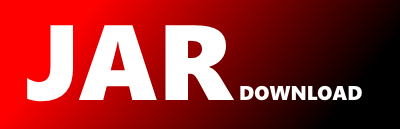
com.kapeta.spring.cloudbucket.AbstractCloudBucketConfig Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cloud-bucket Show documentation
Show all versions of cloud-bucket Show documentation
Cloud bucket support for Kapeta Spring Boot SDK
/*
* Copyright 2023 Kapeta Inc.
* SPDX-License-Identifier: MIT
*/
package com.kapeta.spring.cloudbucket;
import com.kapeta.spring.config.providers.KapetaConfigurationProvider;
import com.kapeta.spring.config.providers.types.ResourceInfo;
import io.minio.*;
import lombok.extern.slf4j.Slf4j;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.context.annotation.Bean;
import java.util.Optional;
/**
* Abstract base class for configuring a cloud bucket using Minio.
* Provides a common configuration structure for interacting with a Minio server and creating a bucket.
*/
@Slf4j
abstract public class AbstractCloudBucketConfig {
private static final String RESOURCE_TYPE = "kapeta/resource-type-cloud-bucket";
private static final String PORT_TYPE = "http";
@Autowired
private KapetaConfigurationProvider configurationProvider;
private final String resourceName;
private final String bucketName;
private final boolean isDebug = System.getenv("DEBUG") != null;
@Value("${minio.url:http://localhost:9000}")
private String minioUrl;
@Value("${minio.username:minioadmin}")
private String defaultUsername;
@Value("${minio.password:minioadmin}")
private String defaultPassword;
/**
* Constructs an instance of the AbstractCloudBucketConfig with the specified resource and bucket name.
*
* @param resourceName The name of the resource associated with the cloud bucket.
* @param bucketName The name of the cloud bucket.
*/
public AbstractCloudBucketConfig(String resourceName, String bucketName) {
this.resourceName = resourceName;
this.bucketName = bucketName;
}
/**
* Configures and provides a MinioClient bean for interacting with a Minio server.
*
* @return Configured MinioClient instance.
* @throws Exception If there is an issue creating the MinioClient.
*/
@Bean
public MinioClient minioClient() throws Exception {
final ResourceInfo info = configurationProvider.getResourceInfo(RESOURCE_TYPE, PORT_TYPE, resourceName);
String minioUsername = Optional.ofNullable(info.getCredentials().get("username")).orElse(defaultUsername);
String minioPassword = Optional.ofNullable(info.getCredentials().get("password")).orElse(defaultPassword);
String protocol = Optional.ofNullable(info.getProtocol()).orElse("http");
minioUrl = String.format("%s://%s:%s", protocol, info.getHost(), info.getPort());
if(isDebug) {
log.info("Resource Name: {}", resourceName);
log.info("Info Resource Name: {}", info.getResource());
log.info("Bucket Name: {}", getBucketName());
log.info("Protocol: {}", protocol);
log.info("Host: {}", info.getHost());
log.info("Port: {}", info.getPort());
log.info("Minio URL: {}", minioUrl);
log.info("Minio Username: {}", minioUsername);
log.info("Minio Password: {}", minioPassword);
}
var builder = MinioClient.builder().endpoint(minioUrl);
if (minioUsername != null && minioPassword != null) {
builder.credentials(minioUsername, minioPassword);
}
MinioClient client = builder.build();
if (!client.bucketExists(BucketExistsArgs.builder().bucket(getBucketName()).build())) {
client.makeBucket(
MakeBucketArgs
.builder()
.bucket(getBucketName())
.build()
);
}
return client;
}
/**
* Provides the name of the cloud bucket.
* If the resource name is provided, the name of the cloud bucket will be retrieved from the Kapeta configuration.
* Otherwise, the name of the cloud bucket will be retrieved from the bucketName field.
* We need to do this because the bucket name is not always the same as the resource name, this is the case when
* the resource is running in clouds like AWS or GCP where the bucket name is a global resource that has to be
* unique across the entire cloud. Here the deployment targets will usually generate a random name for the bucket
* and the resource name will be used to identify the bucket.
*
* @return The name of the cloud bucket.
*/
public String getBucketName() {
final ResourceInfo info = configurationProvider.getResourceInfo(RESOURCE_TYPE, PORT_TYPE, resourceName);
return Optional.ofNullable(info.getResource()).orElse(this.bucketName);
}
/**
* Provides a GetObjectArgs.Builder for retrieving an object from a bucket.
*
* @return GetObjectArgs.Builder instance.
* @throws Exception If there is an issue creating the GetObjectArgs.Builder.
*/
public GetObjectArgs.Builder GetObjectBuilder() throws Exception {
return GetObjectArgs.builder().bucket(getBucketName());
}
/**
* Provides a PutObjectArgs.Builder for storing an object in a bucket.
*
* @return PutObjectArgs.Builder instance.
* @throws Exception If there is an issue creating the PutObjectArgs.Builder.
*/
public PutObjectArgs.Builder PutObjectBuilder() throws Exception {
return PutObjectArgs.builder().bucket(getBucketName());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy