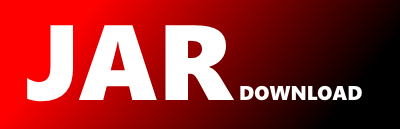
com.kapil.framework.email.BaseEmailDispatcher Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of iframework Show documentation
Show all versions of iframework Show documentation
This is a set of utilities and classes that I have found useful over the years.
In my career spanning over a decade, I have time and again written the same code or
some part of the code over and over again. I never found the time to collate the details
in a reusable library. This project will be a collection of such files.
The work that I have been doing is more than 5 years old, however the project has been
conceived in 2011.
The newest version!
/**
* File name: AbstractEmailProcessor.java Author: Sapient Corporation Creation date: Jun 12, 2007
*/
package com.kapil.framework.email;
import java.util.Date;
import org.apache.commons.mail.Email;
import org.apache.commons.mail.EmailException;
import org.apache.commons.mail.HtmlEmail;
import org.apache.commons.mail.SimpleEmail;
/**
* Provides common information required for sending emails.
*/
public abstract class BaseEmailDispatcher implements IEmailDispatcher
{
private EmailServer server;
public BaseEmailDispatcher(EmailServer server)
{
this.server = server;
}
/**
* Sends an email. Based on the email type, i.e. plaintext or HTML, it creates an appropriate commons-email object.
*
* @param emailContent An {@link EmailVO} object containing information about the email to be sent.
*/
protected void sendEmail(EmailVO emailContent) throws EmailException
{
Email email = null;
// Create an HTML or plaintext email depending on the requirements.
String emailMimeType = emailContent.getMimeType();
if (EmailVO.MimeTypes.HTML.equals(emailMimeType))
{
email = new HtmlEmail();
((HtmlEmail) email).setHtmlMsg(emailContent.getContent());
}
else if (EmailVO.MimeTypes.TEXT.equals(emailMimeType))
{
email = new SimpleEmail();
email.setMsg(emailContent.getContent());
}
email.setHostName(server.getHost());
email.setSmtpPort(server.getPort());
email.setAuthentication(server.getUsername(), server.getPassword());
email.setSSL(server.getUseSSL());
email.setSubject(emailContent.getSubject());
for (String address : emailContent.getFromAddress())
{
email.setFrom(address);
}
for (String address : emailContent.getToAddress())
{
email.addTo(address);
}
email.setSentDate(new Date());
email.send();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy