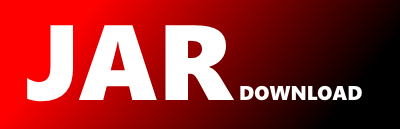
org.mgnlconfig.yaml.dependency.JcrNodeConfigDependency Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of light-yaml Show documentation
Show all versions of light-yaml Show documentation
Enhances extension capabilities of Yaml configuration files (~ to JCR 'extends' property),
provides connection to the JCR config workspace and to all the registries as well
The newest version!
/**
* This file Copyright (c) 2016 Magnolia International
* Ltd. (http://www.magnolia-cms.com). All rights reserved.
*
*
* This file is dual-licensed under both the Magnolia
* Network Agreement and the GNU General Public License.
* You may elect to use one or the other of these licenses.
*
* This file is distributed in the hope that it will be
* useful, but AS-IS and WITHOUT ANY WARRANTY; without even the
* implied warranty of MERCHANTABILITY or FITNESS FOR A
* PARTICULAR PURPOSE, TITLE, or NONINFRINGEMENT.
* Redistribution, except as permitted by whichever of the GPL
* or MNA you select, is prohibited.
*
* 1. For the GPL license (GPL), you can redistribute and/or
* modify this file under the terms of the GNU General
* Public License, Version 3, as published by the Free Software
* Foundation. You should have received a copy of the GNU
* General Public License, Version 3 along with this program;
* if not, write to the Free Software Foundation, Inc., 51
* Franklin St, Fifth Floor, Boston, MA 02110-1301 USA.
*
* 2. For the Magnolia Network Agreement (MNA), this file
* and the accompanying materials are made available under the
* terms of the MNA which accompanies this distribution, and
* is available at http://www.magnolia-cms.com/mna.html
*
* Any modifications to this file must keep this entire header
* intact.
*
*/
package org.mgnlconfig.yaml.dependency;
import info.magnolia.context.Context;
import info.magnolia.jcr.decoration.ContentDecorator;
import info.magnolia.jcr.iterator.FilteringNodeIterator;
import info.magnolia.jcr.iterator.FilteringPropertyIterator;
import info.magnolia.jcr.predicate.AbstractPredicate;
import info.magnolia.jcr.util.NodeTypes;
import info.magnolia.jcr.util.PropertyUtil;
import info.magnolia.jcr.wrapper.ExtendingNodeWrapper;
import info.magnolia.repository.RepositoryConstants;
import java.util.Collections;
import java.util.Map;
import javax.jcr.Node;
import javax.jcr.NodeIterator;
import javax.jcr.Property;
import javax.jcr.PropertyIterator;
import javax.jcr.RepositoryException;
import com.google.common.collect.Maps;
public class JcrNodeConfigDependency implements ConfigDependency {
private final String nodePath;
private final Context context;
private long lastResolved = -1;
public JcrNodeConfigDependency(String nodePath, Context context) {
this.nodePath = nodePath;
this.context = context;
}
@Override
public boolean needsUpdate() {
try {
return context.getJCRSession(RepositoryConstants.CONFIG).getNode(nodePath).getProperty("mgnl:lastResolved").getDate().getTimeInMillis() > lastResolved;
} catch (RepositoryException e) {
return true;
}
}
@Override
public Object getRawData() {
try {
lastResolved = System.currentTimeMillis();
final Node node = context.getJCRSession(RepositoryConstants.CONFIG).getNode(nodePath);
return toMap(node);
} catch (RepositoryException e) {
return Collections.emptyMap();
}
}
private Map toMap(Node node) {
node = new ExtendingNodeWrapper(node);
final Map result = Maps.newHashMap();
try {
final PropertyIterator it = new FilteringPropertyIterator(node.getProperties(), new AbstractPredicate() {
@Override
public boolean evaluateTyped(Property t) {
try {
return !(t.getName().startsWith(NodeTypes.JCR_PREFIX) || t.getName().startsWith(NodeTypes.MGNL_PREFIX));
} catch (RepositoryException e) {
return false;
}
}
}, (ContentDecorator) null);
while (it.hasNext()) {
Property p = it.nextProperty();
Object val = PropertyUtil.getValueObject(p.getValue());
if (val != null) {
result.put(p.getName(), val);
}
}
final NodeIterator nodes = new FilteringNodeIterator(node.getNodes(), new AbstractPredicate() {
@Override
public boolean evaluateTyped(Node t) {
try {
return !(t.getName().startsWith(NodeTypes.JCR_PREFIX) || t.isNodeType(NodeTypes.MetaData.NAME));
} catch (RepositoryException e) {
return false;
}
}
});
while (nodes.hasNext()) {
final Node child = nodes.nextNode();
final Map childMap = toMap(child);
result.put(child.getName(), childMap);
}
return result;
} catch (RepositoryException e) {
return Collections.emptyMap();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy