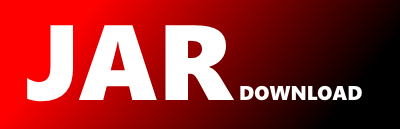
com.katalon.testops.model.CircleCIAgentResource Maven / Gradle / Ivy
/*
* Katalon TestOps API reference
* No description provided (generated by Swagger Codegen https://github.com/swagger-api/swagger-codegen)
*
* OpenAPI spec version: 1.0.0
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*/
package com.katalon.testops.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonValue;
import io.swagger.v3.oas.annotations.media.Schema;
/**
* CircleCIAgentResource
*/
public class CircleCIAgentResource {
@JsonProperty("id")
private Long id = null;
@JsonProperty("name")
private String name = null;
@JsonProperty("url")
private String url = null;
@JsonProperty("username")
private String username = null;
@JsonProperty("token")
private String token = null;
@JsonProperty("project")
private String project = null;
@JsonProperty("vcsType")
private String vcsType = null;
@JsonProperty("branch")
private String branch = null;
@JsonProperty("teamId")
private Long teamId = null;
@JsonProperty("apiKey")
private String apiKey = null;
public CircleCIAgentResource id(Long id) {
this.id = id;
return this;
}
/**
* Get id
* @return id
**/
@Schema(description = "")
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public CircleCIAgentResource name(String name) {
this.name = name;
return this;
}
/**
* Get name
* @return name
**/
@Schema(description = "")
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public CircleCIAgentResource url(String url) {
this.url = url;
return this;
}
/**
* Get url
* @return url
**/
@Schema(description = "")
public String getUrl() {
return url;
}
public void setUrl(String url) {
this.url = url;
}
public CircleCIAgentResource username(String username) {
this.username = username;
return this;
}
/**
* Get username
* @return username
**/
@Schema(description = "")
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
public CircleCIAgentResource token(String token) {
this.token = token;
return this;
}
/**
* Get token
* @return token
**/
@Schema(description = "")
public String getToken() {
return token;
}
public void setToken(String token) {
this.token = token;
}
public CircleCIAgentResource project(String project) {
this.project = project;
return this;
}
/**
* Get project
* @return project
**/
@Schema(description = "")
public String getProject() {
return project;
}
public void setProject(String project) {
this.project = project;
}
public CircleCIAgentResource vcsType(String vcsType) {
this.vcsType = vcsType;
return this;
}
/**
* Get vcsType
* @return vcsType
**/
@Schema(description = "")
public String getVcsType() {
return vcsType;
}
public void setVcsType(String vcsType) {
this.vcsType = vcsType;
}
public CircleCIAgentResource branch(String branch) {
this.branch = branch;
return this;
}
/**
* Get branch
* @return branch
**/
@Schema(description = "")
public String getBranch() {
return branch;
}
public void setBranch(String branch) {
this.branch = branch;
}
public CircleCIAgentResource teamId(Long teamId) {
this.teamId = teamId;
return this;
}
/**
* Get teamId
* @return teamId
**/
@Schema(description = "")
public Long getTeamId() {
return teamId;
}
public void setTeamId(Long teamId) {
this.teamId = teamId;
}
public CircleCIAgentResource apiKey(String apiKey) {
this.apiKey = apiKey;
return this;
}
/**
* Get apiKey
* @return apiKey
**/
@Schema(description = "")
public String getApiKey() {
return apiKey;
}
public void setApiKey(String apiKey) {
this.apiKey = apiKey;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
CircleCIAgentResource circleCIAgentResource = (CircleCIAgentResource) o;
return Objects.equals(this.id, circleCIAgentResource.id) &&
Objects.equals(this.name, circleCIAgentResource.name) &&
Objects.equals(this.url, circleCIAgentResource.url) &&
Objects.equals(this.username, circleCIAgentResource.username) &&
Objects.equals(this.token, circleCIAgentResource.token) &&
Objects.equals(this.project, circleCIAgentResource.project) &&
Objects.equals(this.vcsType, circleCIAgentResource.vcsType) &&
Objects.equals(this.branch, circleCIAgentResource.branch) &&
Objects.equals(this.teamId, circleCIAgentResource.teamId) &&
Objects.equals(this.apiKey, circleCIAgentResource.apiKey);
}
@Override
public int hashCode() {
return Objects.hash(id, name, url, username, token, project, vcsType, branch, teamId, apiKey);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class CircleCIAgentResource {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" url: ").append(toIndentedString(url)).append("\n");
sb.append(" username: ").append(toIndentedString(username)).append("\n");
sb.append(" token: ").append(toIndentedString(token)).append("\n");
sb.append(" project: ").append(toIndentedString(project)).append("\n");
sb.append(" vcsType: ").append(toIndentedString(vcsType)).append("\n");
sb.append(" branch: ").append(toIndentedString(branch)).append("\n");
sb.append(" teamId: ").append(toIndentedString(teamId)).append("\n");
sb.append(" apiKey: ").append(toIndentedString(apiKey)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy